Building 5 Stunning Responsive Team Sections with Tailwind CSS and Next.js 14

Creating stunning team sections can be a great way to showcase your team members on your website. With Next.js and Tailwind CSS, we can easily build these sections with clean code and a responsive design.
In this tutorial, we’ll walk through how to create 5 responsive and ready to use team sections using Next.js and Tailwind CSS. We’ll start by setting up our project and installing the necessary dependencies. Then we’ll build out our team section component using React and Tailwind CSS. Finally, we’ll add some styles to make it look good on different devices.
Prerequisites
- Before we begin, make sure you have the following requirements met:
- Basic understanding of Next.js and Tailwind CSS.
- Node.js and npm (or yarn) installed on your machine.
- Basic knowledge of HTML and CSS.
Setting up the Project
To get started, let's create a new Next.js project and install Tailwind CSS. Open your terminal and run the following commands:
npx create-next-app@latest tailwind_teams
cd tailwind_teams
Additional configuration:
I have added the following code to the tailwind config file to center the container:
theme: { container: { center: true, }, }
Adding the Team Data File:
I have added a demo data file for team member details like username, Full Name, Image links and their social media links.
export const profileDetails = [
{
username: 'DevMaster',
fullName: 'Alex Codington',
bio: 'Passionate developer with a knack for turning caffeine into code. Creating digital wonders and solving problems with elegant solutions.',
github: 'https://www.github.com/',
twitter: 'https://twitter.com/',
facebook: 'https://www.facebook.com/',
instagram: 'https://instagram.com/',
background: '/assets/images/bg-1.jpg',
image: '/assets/images/img-1.jpg',
},
{
username: 'CodeExplorer',
fullName: 'Sophie Hackman',
bio: 'Explorer of new technologies and coding realms. Transforming ideas into lines of code. Always in pursuit of the next coding adventure.',
github: 'https://www.github.com/',
twitter: 'https://twitter.com/',
facebook: 'https://www.facebook.com/',
instagram: 'https://instagram.com/',
background: '/assets/images/bg-2.jpg',
image: '/assets/images/img-2.jpg',
},
{
username: 'TechEnthusiast',
fullName: 'Olivia Codefield',
bio: 'Enthusiastic about all things tech. A problem solver with a passion for innovation. Turning ideas into reality through the power of code.',
github: 'https://www.github.com/',
twitter: 'https://twitter.com/',
facebook: 'https://www.facebook.com/',
instagram: 'https://instagram.com/',
image: '/assets/images/img-3.jpg',
background: '/assets/images/bg-3.jpg',
},
{
username: 'ByteWizard',
fullName: 'Ethan Techsmith',
bio: 'Magician of bytes and bytes. Crafting magic with algorithms and data structures. Making the digital world a better place, one line at a time.',
github: 'https://www.github.com/',
twitter: 'https://twitter.com/',
facebook: 'https://www.facebook.com/',
instagram: 'https://instagram.com/',
background: '/assets/images/bg-4.jpg',
image: '/assets/images/img-4.jpg',
},
{
username: 'CyberNinja',
fullName: 'Max Secureblade',
bio: 'Cybersecurity ninja with a mission to protect the digital realm. Expert in securing networks and defeating cyber threats. Silent, but deadly in code battles.',
github: 'https://www.github.com/',
twitter: 'https://twitter.com/',
facebook: 'https://www.facebook.com/',
instagram: 'https://instagram.com/',
image: '/assets/images/img-1.jpg',
background: '/assets/images/bg-1.jpg',
},
{
username: 'UIUXGuru',
fullName: 'Lily Designsmith',
bio: 'UI/UX design guru with a keen eye for aesthetics and user experience. Crafting beautiful and intuitive interfaces that leave a lasting impression.',
github: 'https://www.github.com/',
twitter: 'https://twitter.com/',
facebook: 'https://www.facebook.com/',
instagram: 'https://instagram.com/',
image: '/assets/images/img-2.jpg',
background: '/assets/images/bg-2.jpg',
},
];
Creating Team Section and styling with Tailwind CSS:
You can check out this video for styling the team section.
You can find the complete code for this tutorial on our GitHub repository.
We hope you found this tutorial helpful and that it has inspired you to create beautiful team sections for your own website. Happy coding!
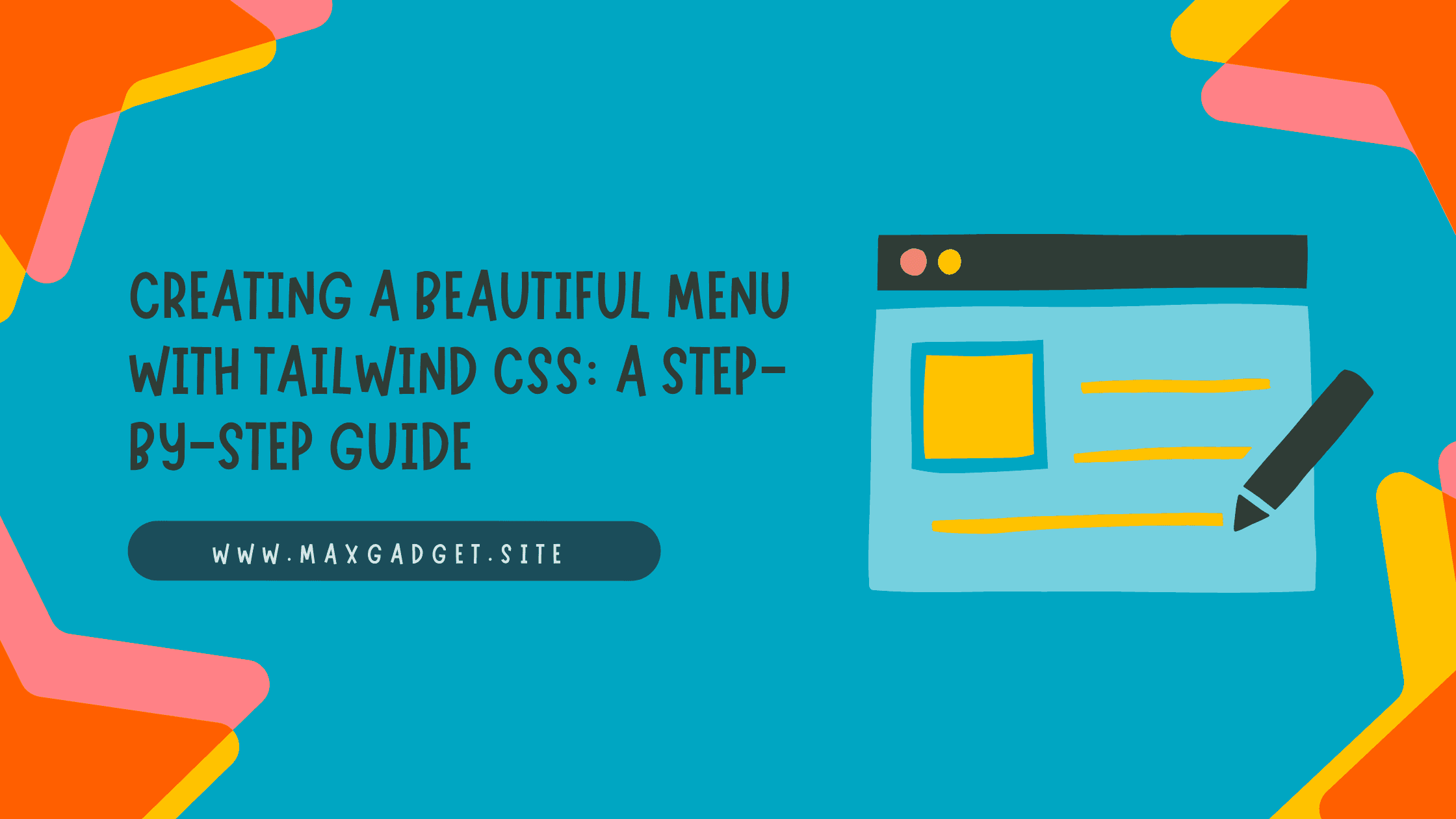
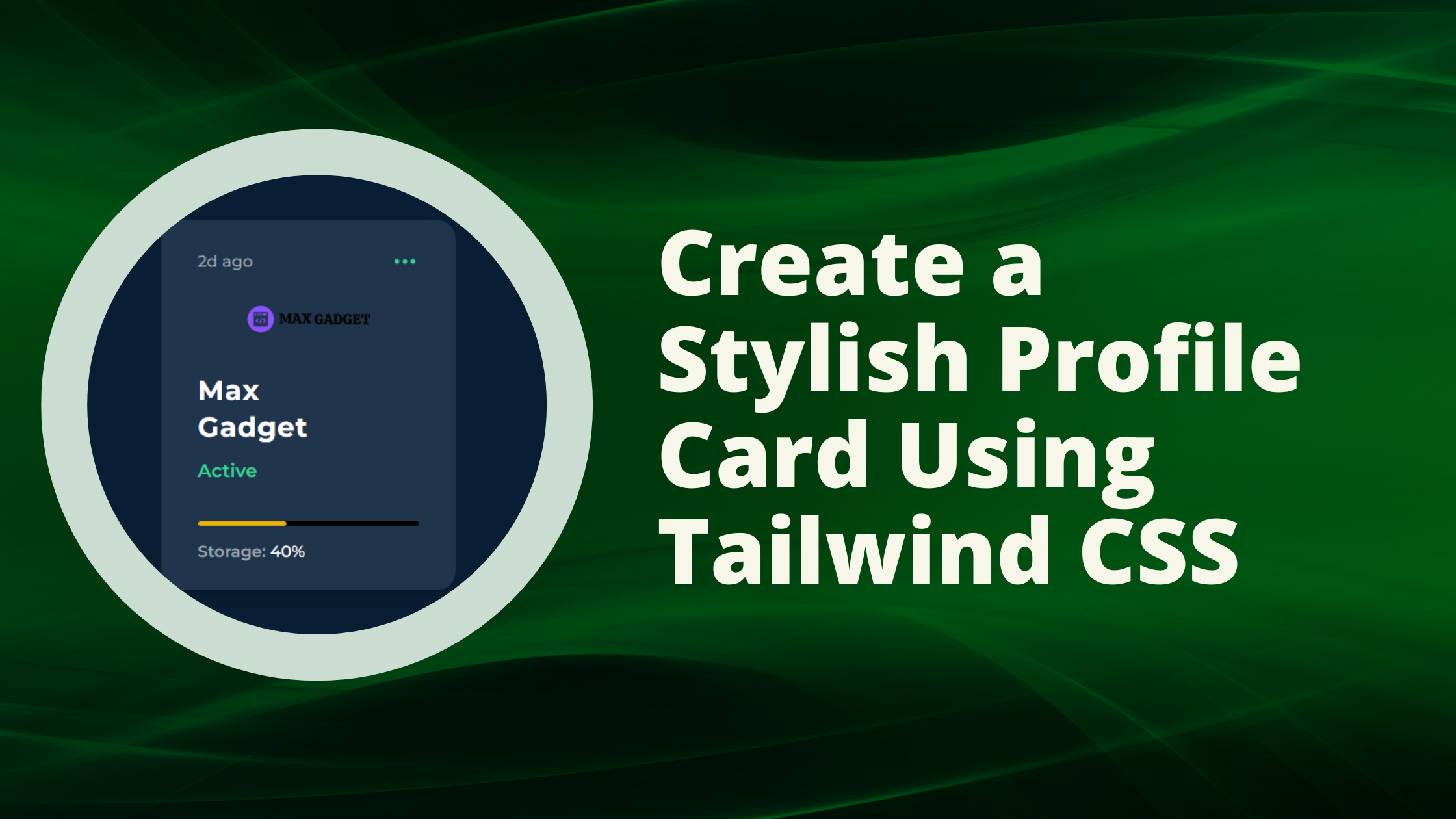
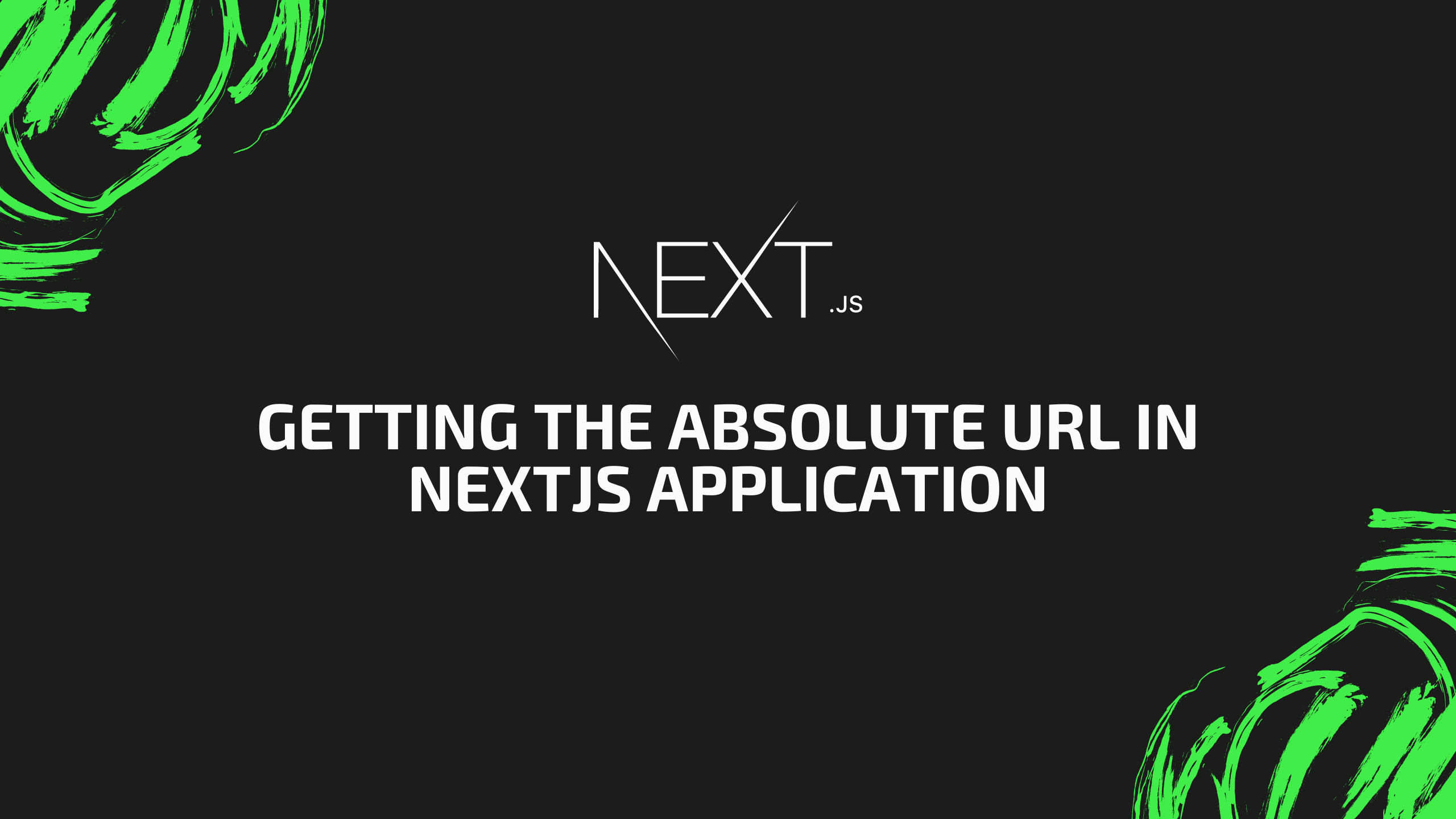
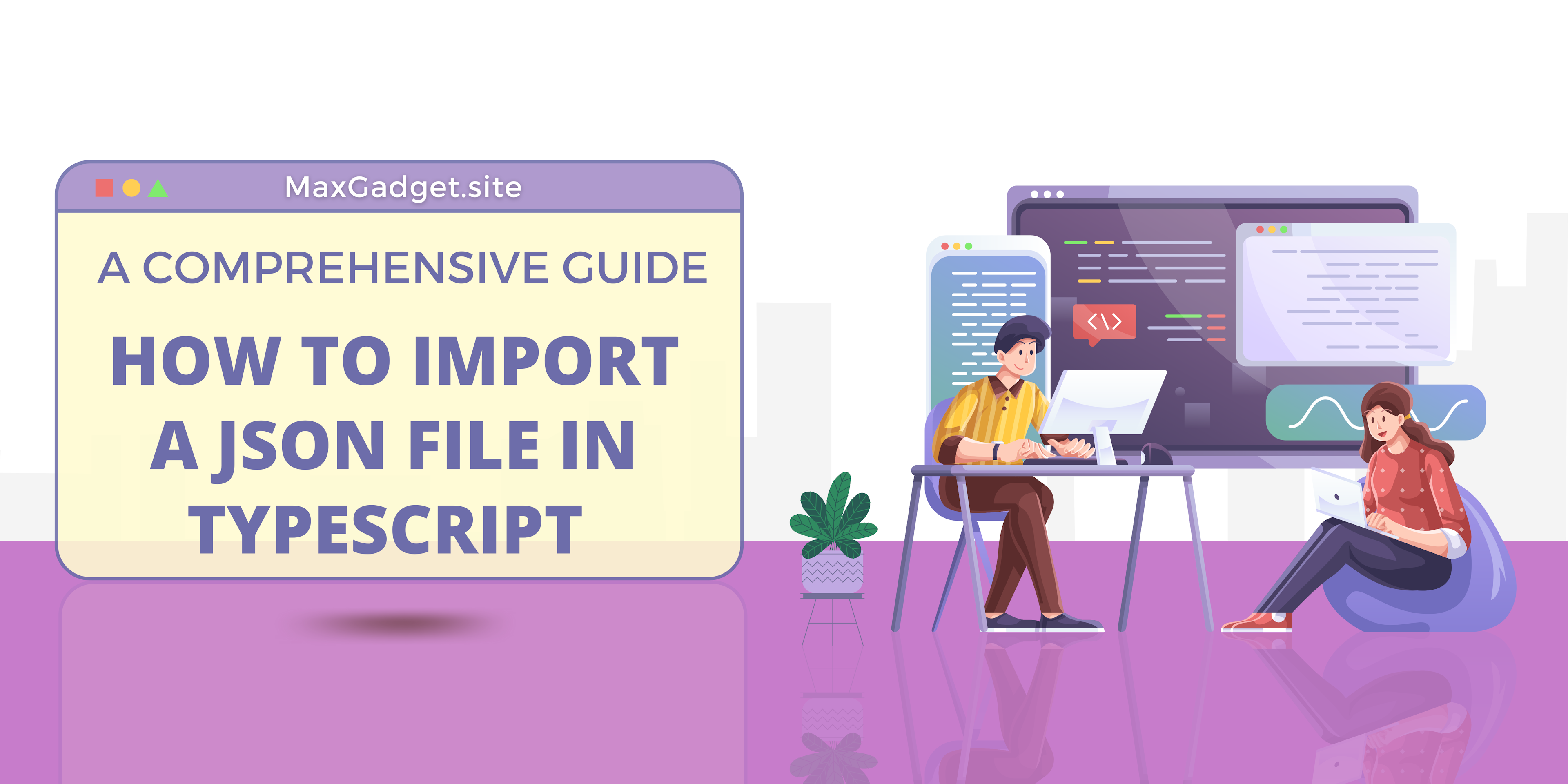
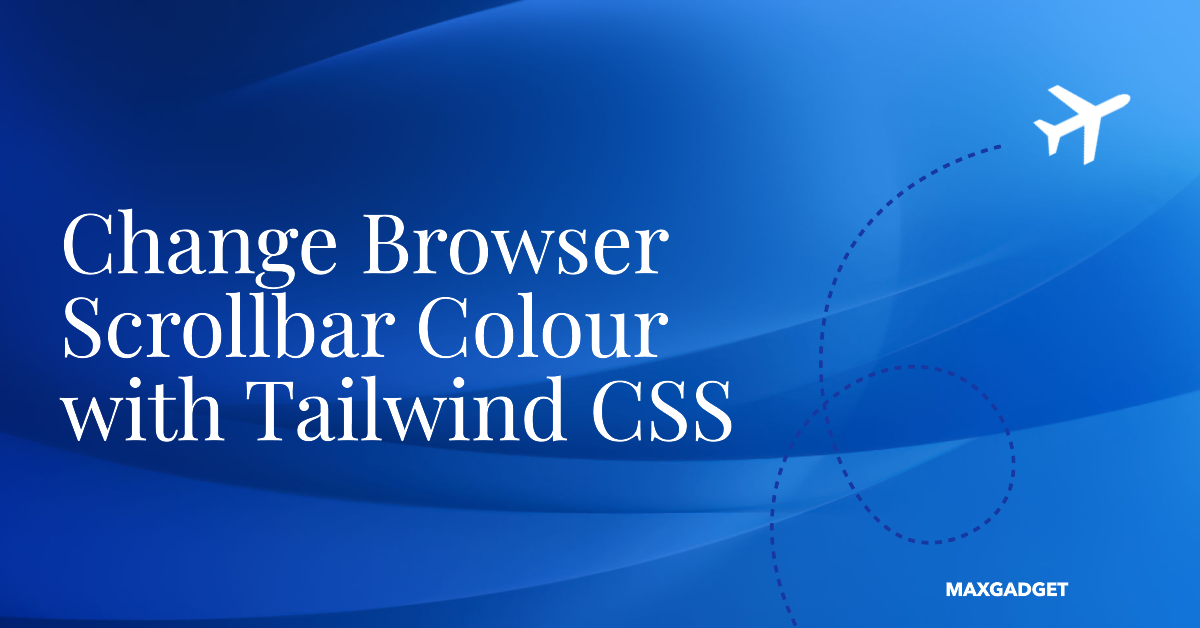
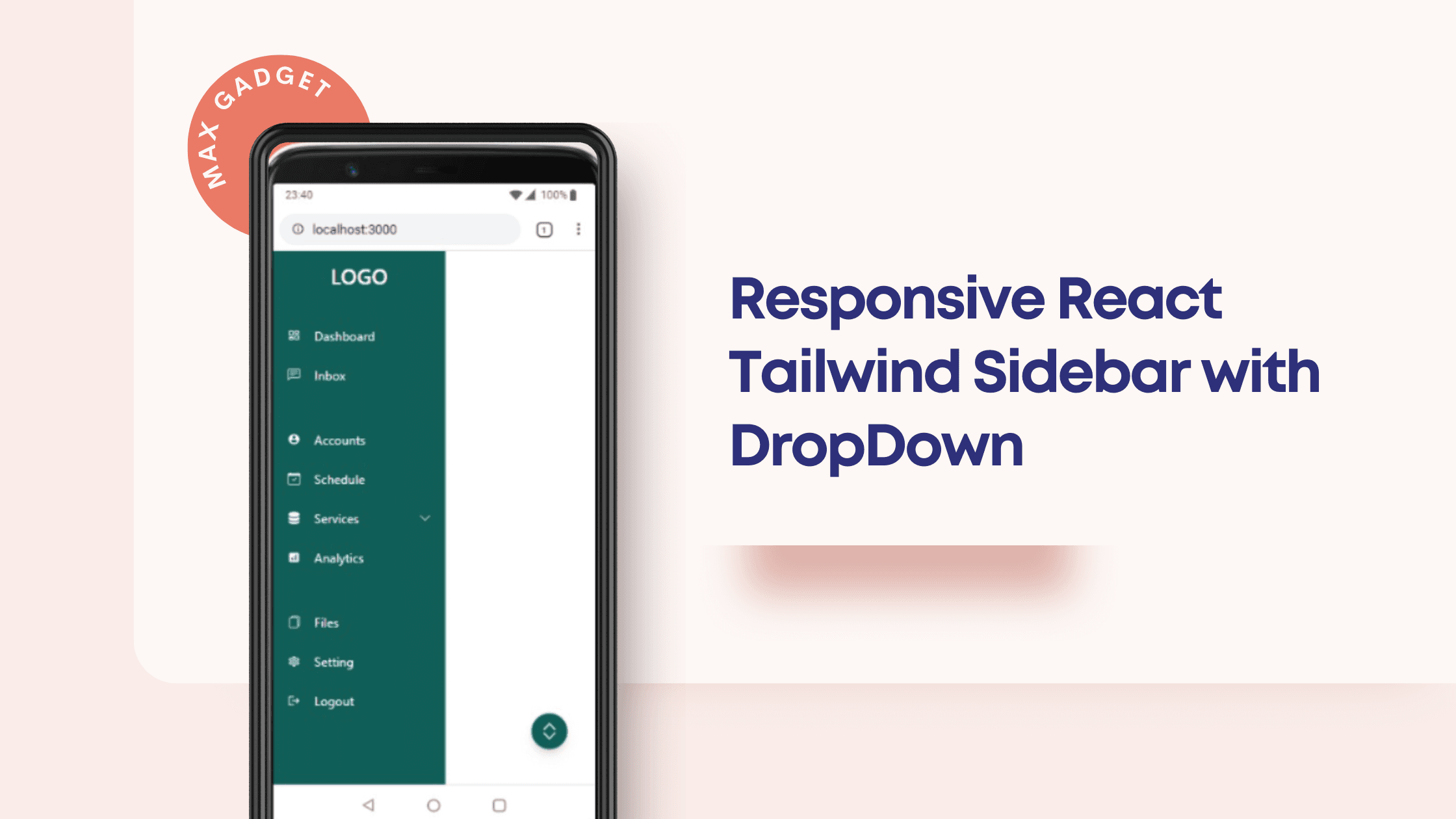