How to Change Browser Scrollbar Color with Tailwind CSS

Styling the scrollbar of a web page can be a tricky task, as the scrollbar is not an element that we can style directly with CSS. However, it is possible to change the scrollbar color with a little bit of trickery.
One approach is to use a library like jQuery.scrollbar, which allows you to customize the scrollbar's appearance with CSS. However, if you're using a utility-first CSS framework like Tailwind CSS, you might be looking for a more lightweight solution.
In this article, we'll explore how to change the scrollbar color using Tailwind CSS and a few lines of JavaScript.
Styling the Scrollbar with CSS
To style the scrollbar, we'll need to use a pseudo-element. A pseudo-element is a virtual element that doesn't exist in the DOM, but we can style it with CSS. In this case, we'll use the ::-webkit-scrollbar pseudo-element, which represents the scrollbar in webkit-based browsers (such as Chrome and Safari).
To style the scrollbar with CSS, we can use the following code:
::-webkit-scrollbar {
width: 10px;
height: 10px;
background-color: #f5f5f5;
}
::-webkit-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
In this code, we're setting the width and height of the scrollbar to 10px, and we're giving it a light grey background color. We're also styling the thumb (the part of the scrollbar that you drag) with a black background color and a border radius of 10px.
This code will work in webkit-based browsers, but it won't work in other browsers. To support all modern browsers, we'll need to use vendor prefixes for the pseudo-elements. Here's the updated code:
::-webkit-scrollbar {
width: 10px;
height: 10px;
background-color: #f5f5f5;
}
::-webkit-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
::-moz-scrollbar {
width: 10px;
height: 10px;
background-color: #f5f5f5;
}
::-moz-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
::-ms-scrollbar {
width: 10px;
height: 10px;
background-color: #f5f5f5;
}
::-ms-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
::scrollbar {
width: 10px;
height: 10px;
background-color: #f5f5f5;
}
::scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
This code will work in all modern browsers, but it can be a bit cumbersome to maintain. Fortunately, there's a better way to style the scrollbar using Tailwind CSS.
Styling the Scrollbar with Tailwind CSS
To style the scrollbar with Tailwind CSS, we'll need to use the customForms plugin, which allows us to apply styles to pseudo-elements.
First, we'll need to install the customForms plugin:
npm install @tailwindcss/custom-forms
Then, we'll need to add the plugin to our configuration file:
module.exports = {
theme: {},
variants: {},
plugins: [
require('@tailwindcss/custom-forms')
]
}
With the plugin installed and configured, we can now use the scrollbar-* utilities to style the scrollbar. For example, to give the scrollbar a black background color and a border radius of 10px, we can use the following code:
.scrollbar-black::-webkit-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
.scrollbar-black::-moz-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
.scrollbar-black::-ms-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
.scrollbar-black::scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
This code uses the scrollbar-black utility to give the thumb a black background color and a border radius of 10px.
To apply this style to an element, we can simply add the scrollbar-black class to the element. For example:
<div class="scrollbar-black">
<!-- Content goes here -->
</div>
Tailwind CSS also provides utilities for setting the width and height of the scrollbar, as well as the background color of the track (the part of the scrollbar that the thumb moves on).
Changing the Scrollbar Color with JavaScript
In some cases, you might want to change the scrollbar color dynamically with JavaScript. To do this, we'll need to use a little bit of trickery.
First, we'll need to create a class that contains the styles for the scrollbar. For example:
.scrollbar-black::-webkit-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
.scrollbar-black::-moz-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
.scrollbar-black::-ms-scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
.scrollbar-black::scrollbar-thumb {
background-color: #000000;
border-radius: 10px;
}
Next, we'll need to add a class to the html element that will contain the styles for the scrollbar. We can do this with JavaScript like this:
document.documentElement.classList.add('scrollbar-black');
This code adds the scrollbar-black class to the html element, which applies the styles for the scrollbar.
To remove the scrollbar-black class and revert to the default scrollbar styles, we can use the following code:
document.documentElement.classList.remove('scrollbar-black');
With these two functions, we can easily change the scrollbar color using JavaScript.
For example, let's say we want to change the scrollbar color when a button is clicked. We can do this with the following code:
const button = document.querySelector('button');
button.addEventListener('click', () => {
document.documentElement.classList.toggle('scrollbar-black');
});
This code adds an event listener to the button that toggles the scrollbar-black class on the html element when the button is clicked.
And that's it! With a few lines of CSS and JavaScript, we can change the scrollbar color with Tailwind CSS. I hope this article has been helpful, and please let me know if you have any questions.
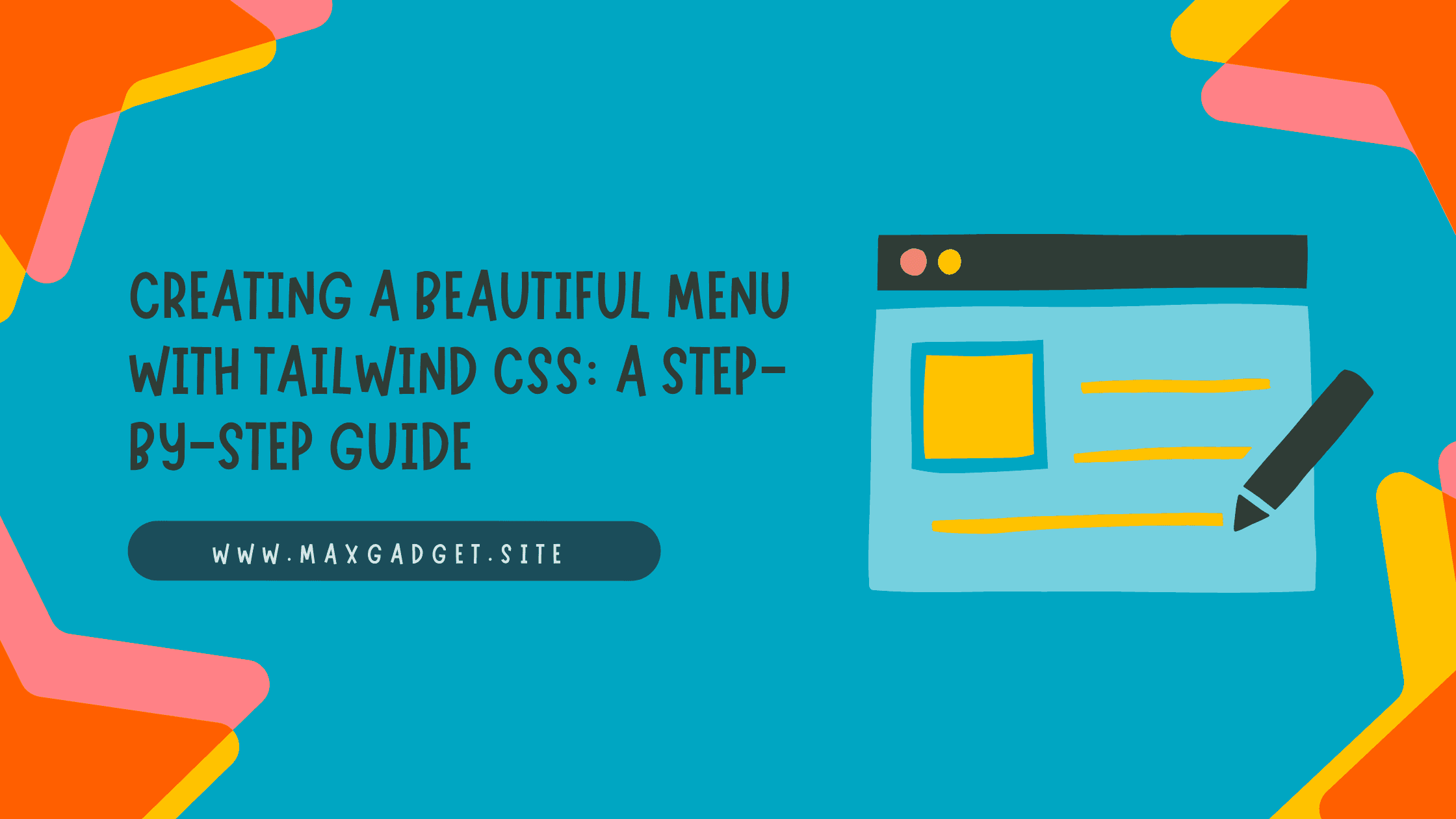
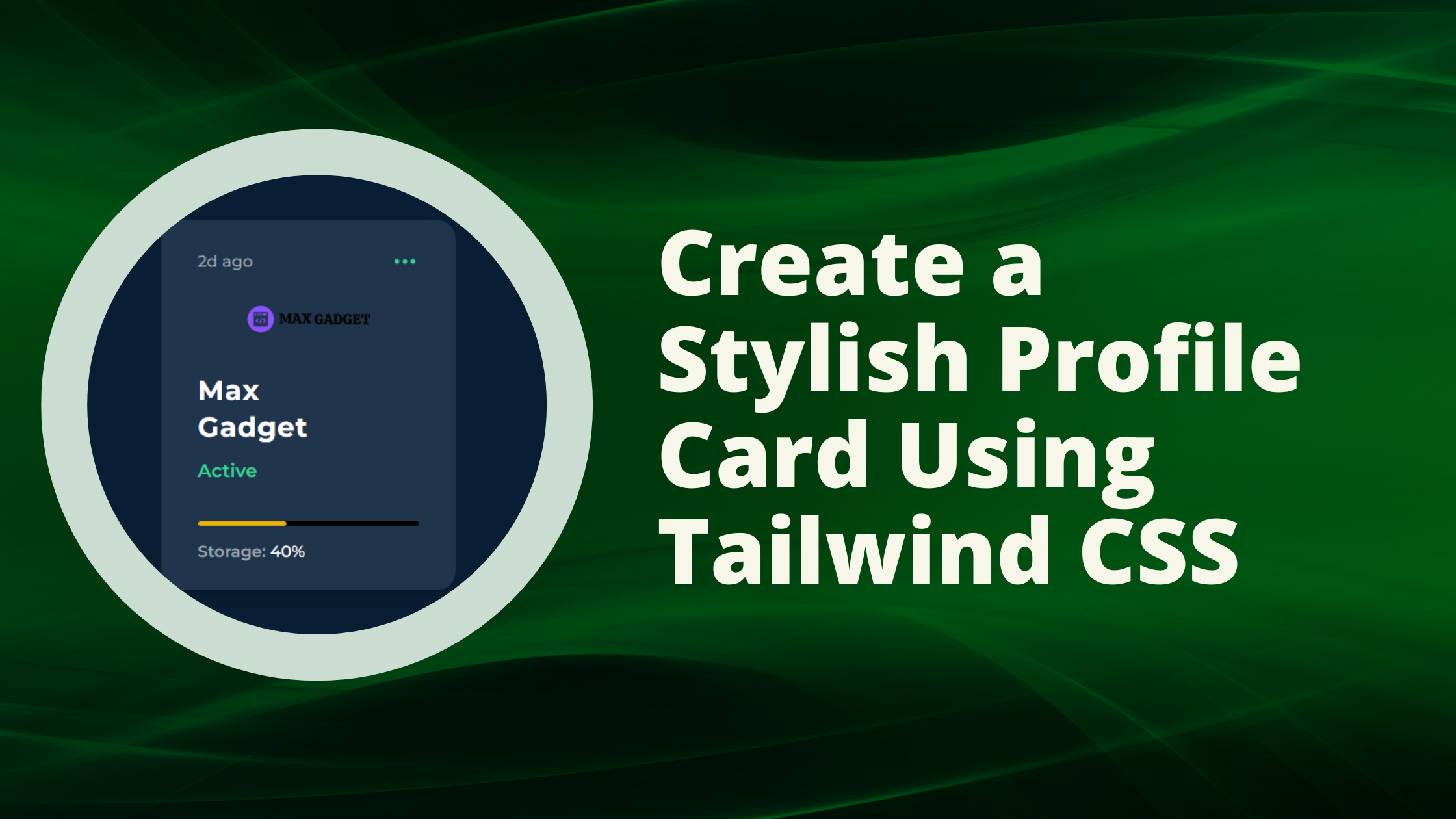
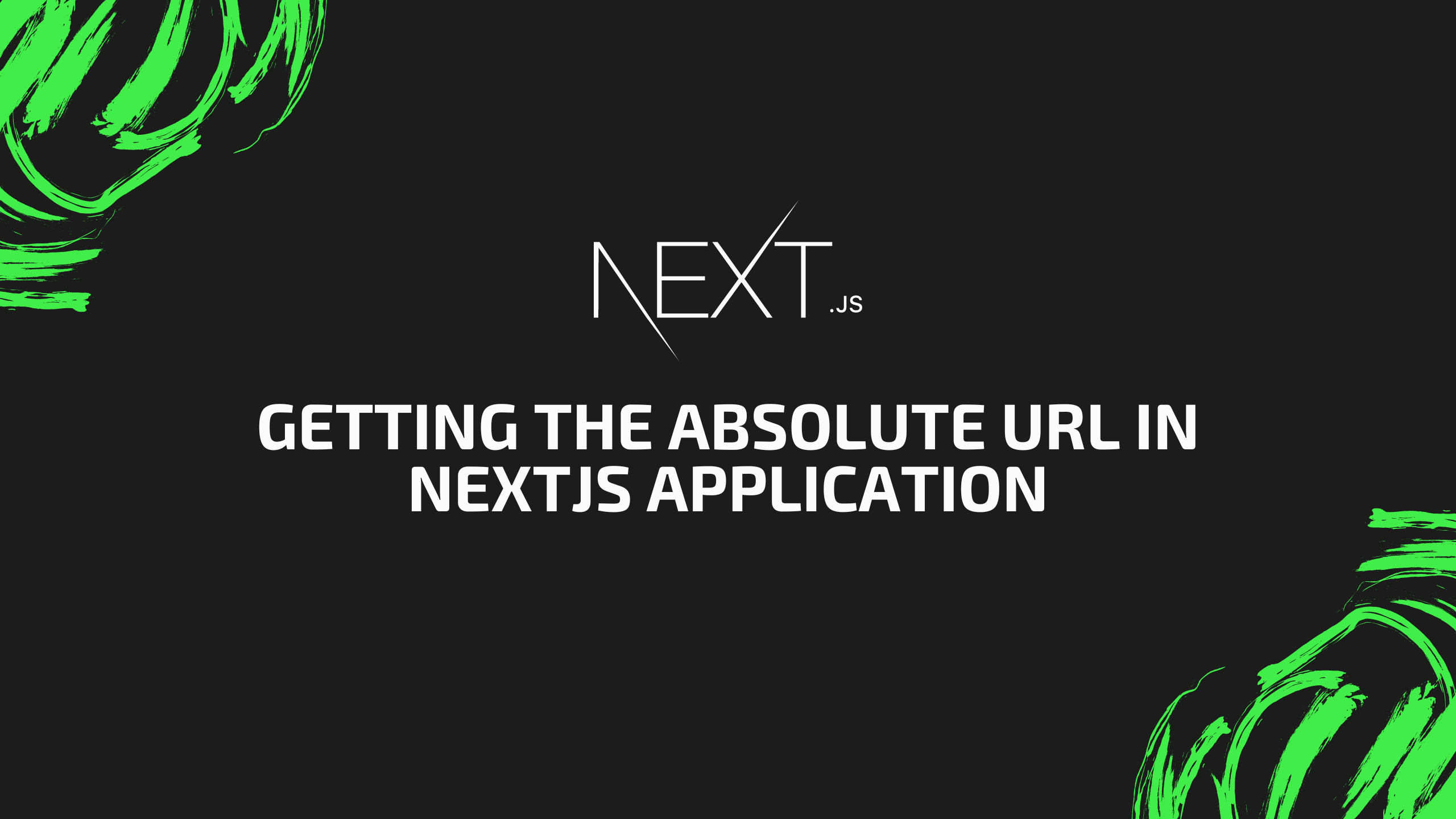
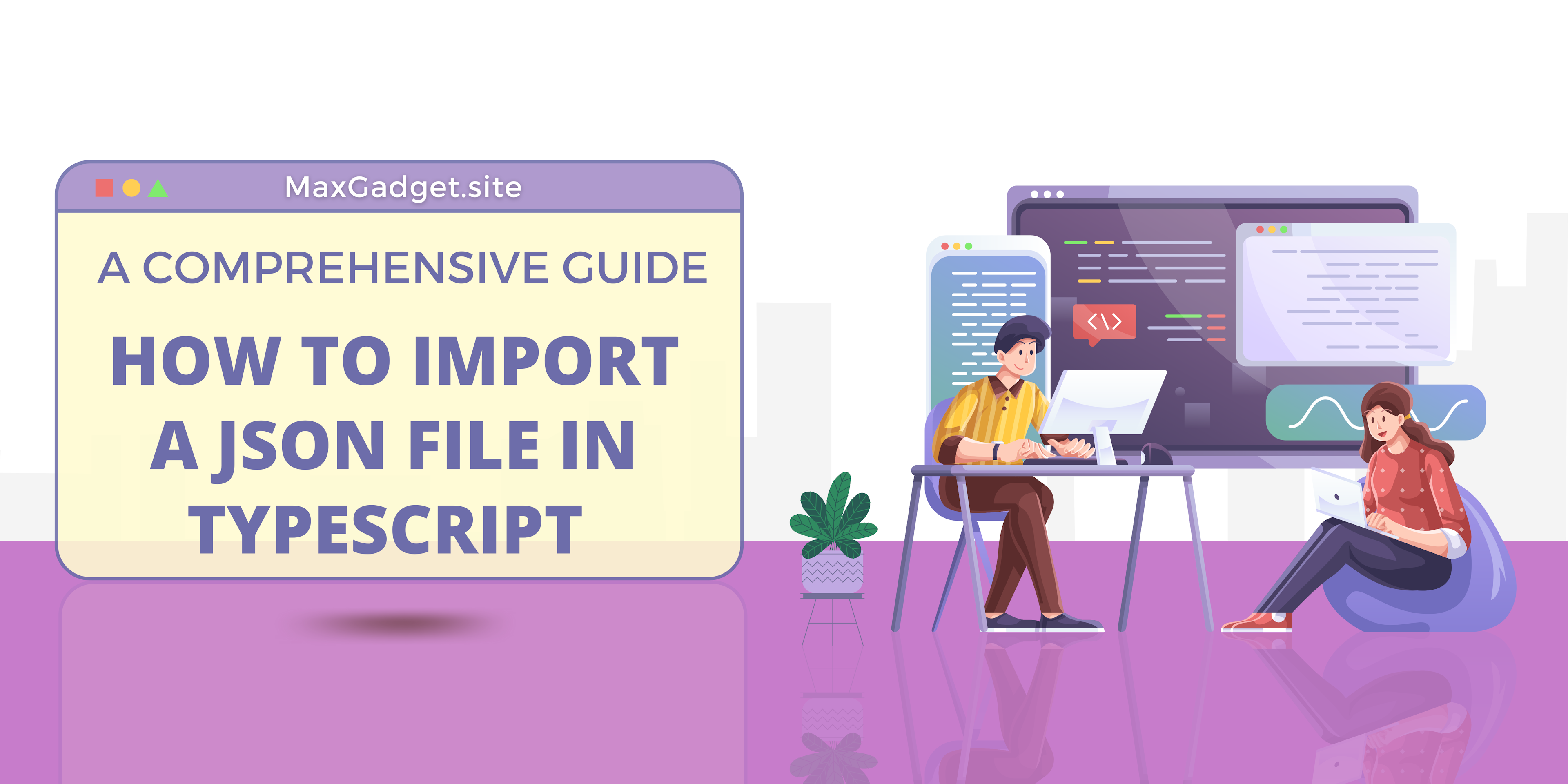
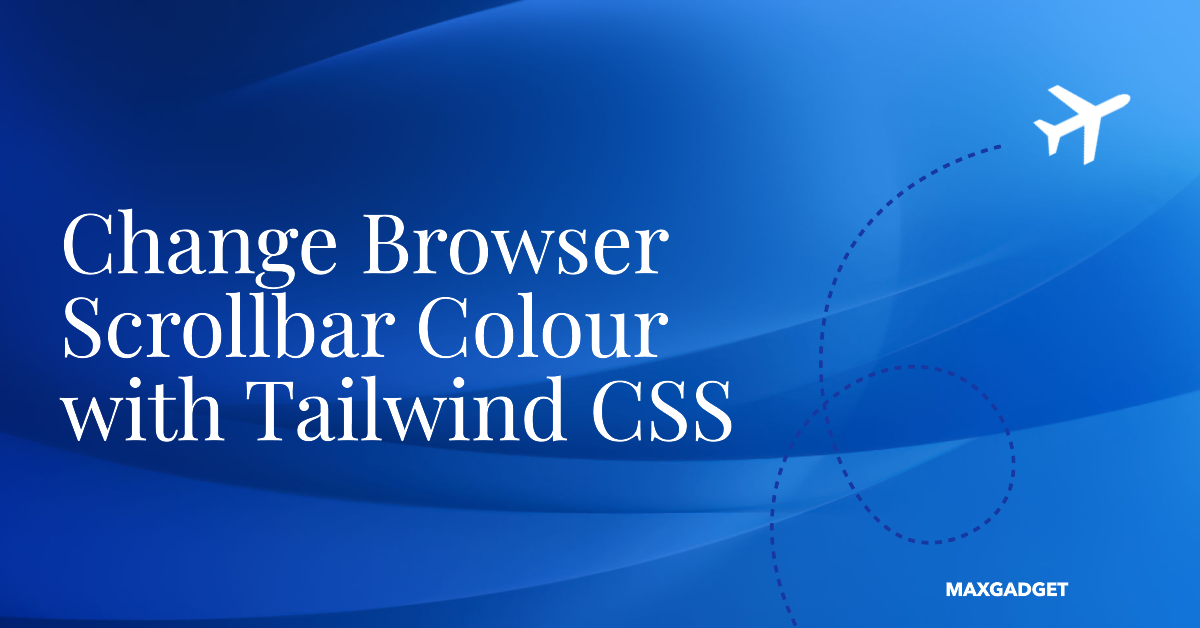
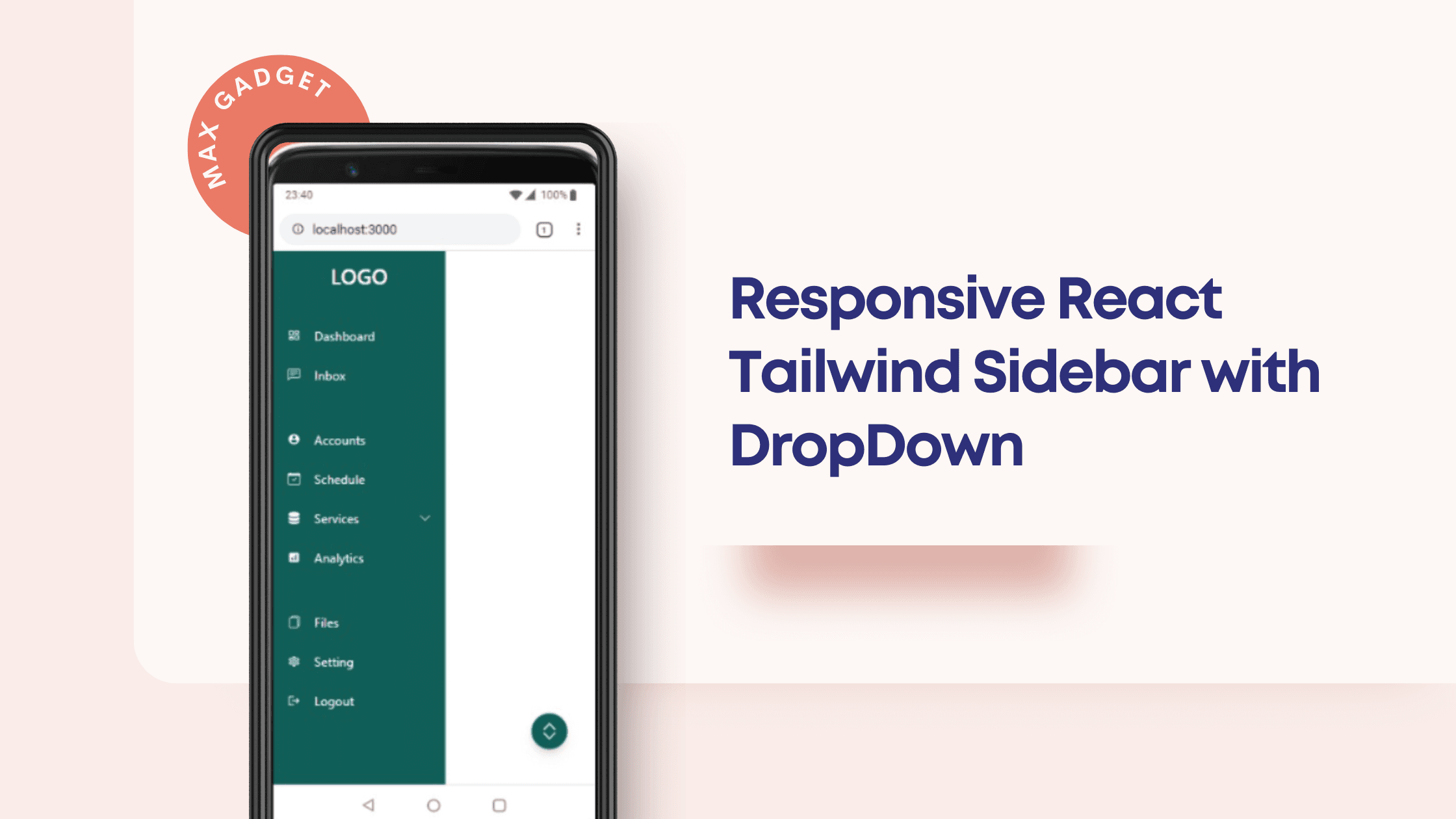