Mastering Next.js: Getting the Absolute URL in Nextjs Application

Next.js is a highly popular framework for building server-rendered React apps. Its powerful routing system, dynamic page rendering, and integration with Node.js offer developers endless possibilities. To ensure better SEO optimization and navigation within the app, obtaining the absolute URL is crucial.
This article provides insights on various techniques to get the absolute URL in Next.js. You will learn about common scenarios, potential pitfalls, and best practices for implementing this essential feature effectively.
Next js get absolute URL: Understanding the Basics
To better understand the importance of an absolute URL in your Next.js application, let's first clarify its definition.
What is an Absolute URL?
An absolute URL includes all necessary components, such as the protocol and domain name, to indicate the exact location of a web resource and enable accurate linking and navigation.
Why is Obtaining the Absolute URL Important in Next.js?
Using Next.js, pages can be rendered on both server and client sides. Absolute URLs ensure the correct page is displayed when sharing links or transitioning between pages, improving visibility and SEO rankings by allowing search engines to accurately index your content.
Now that we have a good grasp of the concept, let's look at various ways to acquire the absolute URL in Next.js.
Server-Side Rendering (SSR) Techniques
There are various methods for obtaining the absolute URL in a server-rendered Next.js application. Let's delve into the most popular techniques for next js get current url server side:
Method 1: Using the req object
With server-side rendering, you can utilize the request (req) object to gain access to the absolute URL. By parsing important data from the headers of said request, constructing a full URL becomes possible. you can see next-absolute-url example here:
import { IncomingMessage } from 'http';
function getAbsoluteUrl(req: IncomingMessage) {
const protocol = req.headers['x-forwarded-proto'] || 'http';
const host = req.headers['host'];
const path = req.url;
return `${protocol}://${host}${path}`;
}
To form the absolute URL, we take out the protocol, host, and path from the request headers and join them together as shown in the previous example.
Method 2: Using Next.js Router
The Router object in Next.js enables simplified URL handling with its powerful router, allowing for easy retrieval of absolute URLs using the asPath property. You can check Next js get absolute url example here:
import { useRouter } from 'next/router';
function getAbsoluteUrl() {
const router = useRouter();
const { asPath } = router;
return `${window.location.origin}${asPath}`;
}
By using the useRouter hook from Next.js, we can access the current route's asPath property and combine it with window.location.origin to retrieve the full URL in this example.
Client-Side Rendering (CSR) Techniques
In a client-side rendering context, obtaining the absolute URL has slightly different options. Let's look at two commonly used techniques for CSR in Next.js.
Method 3: Utilizing the window Object
The current URL can be obtained in a client-side rendered Next.js application by using the window object. you can see next-absolute-url example here:
function getAbsoluteUrl() {
return window.location.href;
}
We can obtain the entire URL of the current page by using the href property of window.location.
Method 4: Leveraging the Next.js Router
You can use the Next.js Router to get the absolute URL in a client-side rendering context. You can check Next js get absolute url example here:
import { useRouter } from 'next/router';
function getAbsoluteUrl() {
const router = useRouter();
const { asPath } = router;
return `${window.location.origin}${asPath}`;
}
Like the SSR technique, we can use the useRouter hook to access the current route's asPath property and get the complete URL when combined with window.location.origin.
Frequently asked questions about "next js get absolute URL"
Why is the absolute URL necessary for SEO optimization?
Having a complete absolute URL is critical for SEO optimization, as it ensures that search engines index your pages correctly. This improves visibility and organic search rankings because search engines can accurately associate your content with the correct domain and path.
Can I use relative URLs instead of absolute URLs in Next.js?
Although relative URLs have limited use, Next.js applications are best served by absolute URLs which guarantee precise navigation and linking across pages, irrespective of location or rendering context.
Are there any potential security risks associated with exposing the absolute URL?
When working with absolute URLs, it's important to consider potential security risks and avoid including sensitive information like session tokens or API keys in the URL string. To ensure secure data transmission, use server-side methods or separate authentication mechanisms instead.
How can I handle dynamic routing and obtain the absolute URL for dynamic pages in Next.js?
In order to achieve dynamic routing and obtain the absolute URL for dynamic pages, you can merge the earlier techniques with Next.js' dynamic routing capabilities. By including required parameters in the URL, you can create URLs that are adaptive to the current page's state and offer precise navigation.
Can I use third-party libraries or packages to simplify obtaining the absolute URL in Next.js?
Third-party libraries and packages can help simplify the process of obtaining absolute URLs in Next.js. One such example is next-url-prettifier, which generates user-friendly URLs that are better for SEO and enhance overall user experience.
How can I ensure my Next.js application's absolute URLs are search engine-friendly?
For better search engine optimization of your Next.js application's absolute URLs, it is advisable to use canonicalization techniques and follow SEO best practices. Descriptive, concise URLs and optimized metadata along with proper internal linking can help prevent issues related to duplicated content.
Conclusion
To maximize your Next.js application's performance, user experience, and SEO, obtaining the absolute URL is essential. In this article, we explored various techniques to achieve this in both server-side rendering (SSR) and client-side rendering (CSR) contexts. By utilizing methods like accessing the req object, using the Next.js Router, and leveraging the window object for navigation accuracy and linking, you can ensure optimized functionality.
However, it's crucial to prioritize security implications and maintain SEO best practices when working with absolute URLs. Implementing these approaches while adhering to best practices equips you with optimal tools for your Next.js application development endeavors.
In addition to the FAQs listed above, it's worth noting that obtaining absolute URLs in Next.js can also be beneficial for tracking purposes. With precise URLs, you can track user behavior, pageviews, and other metrics with greater accuracy. This can help you identify areas of your application that need improvement and optimize user engagement.
Furthermore, obtaining absolute URLs can be useful for creating shareable links. With clear, understandable links, users can easily share your application's content with others, leading to increased traffic and engagement.
It's also worth considering that obtaining absolute URLs may require different approaches depending on the deployment environment. For example, on platforms like Vercel, certain server-side methods for obtaining absolute URLs may not be available.
Overall, obtaining absolute URLs in Next.js is a critical aspect of application development. By understanding the FAQs outlined above and utilizing appropriate techniques, you can ensure optimal functionality, improve SEO optimization, and enhance user experience.
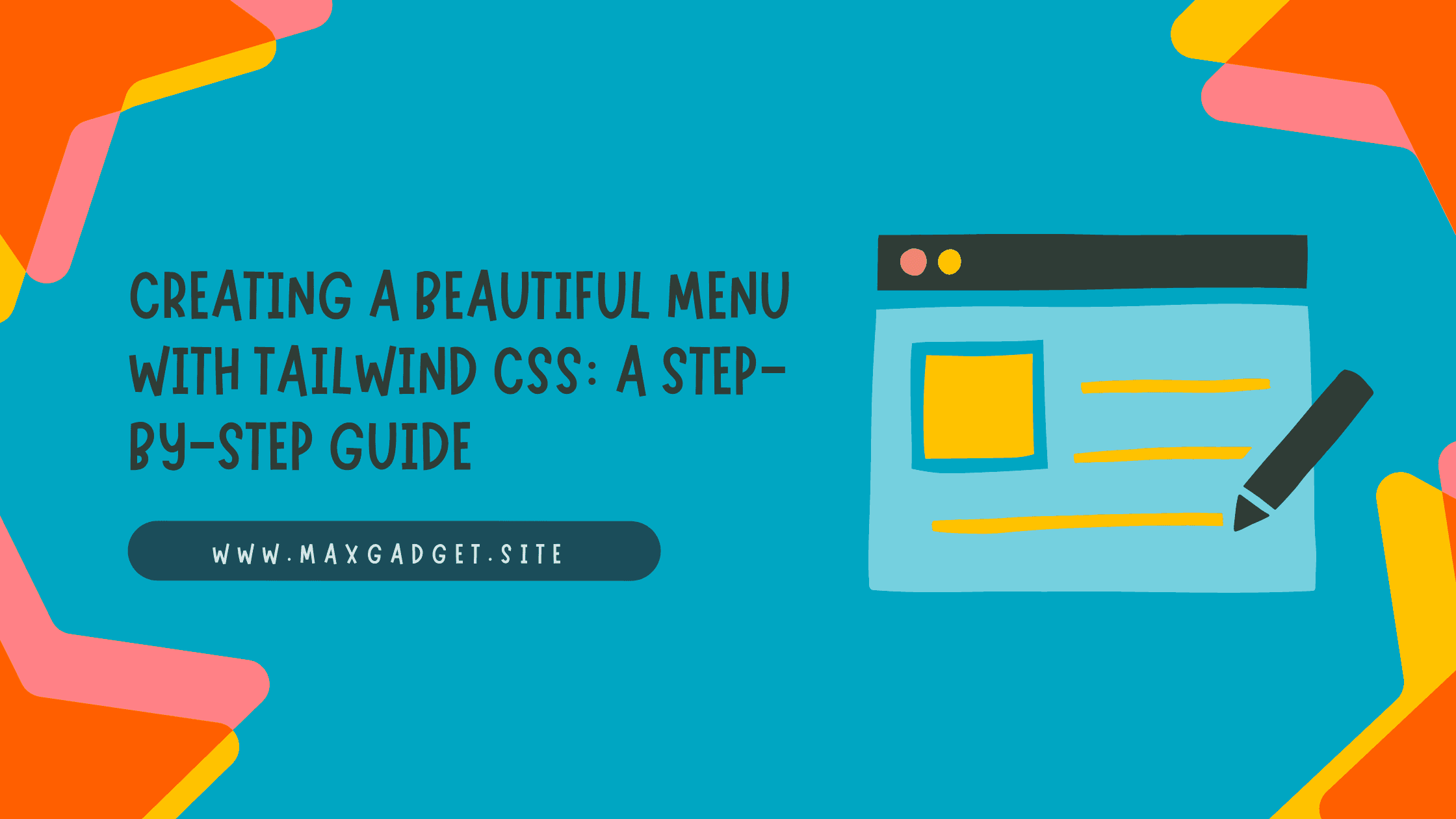
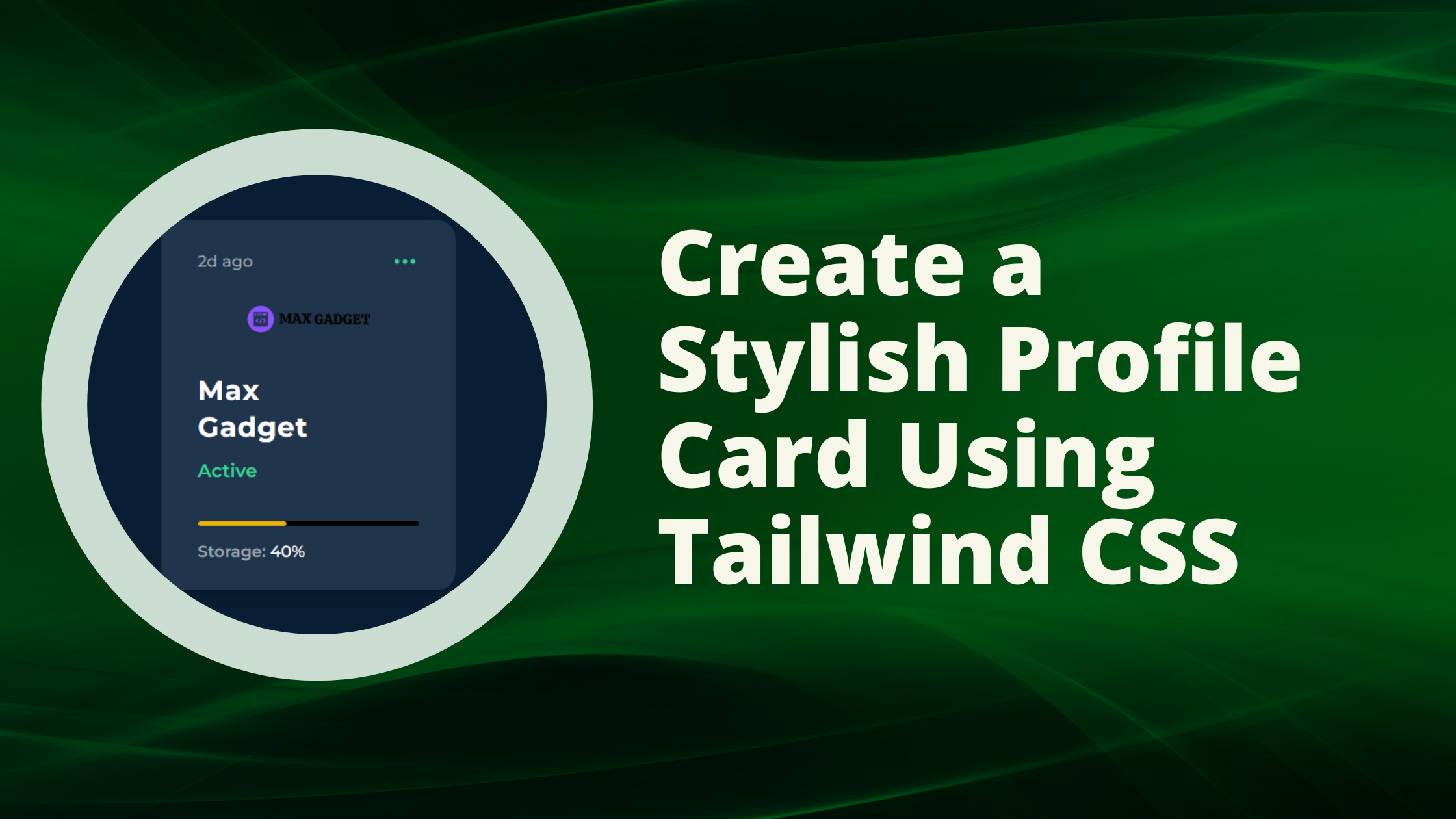
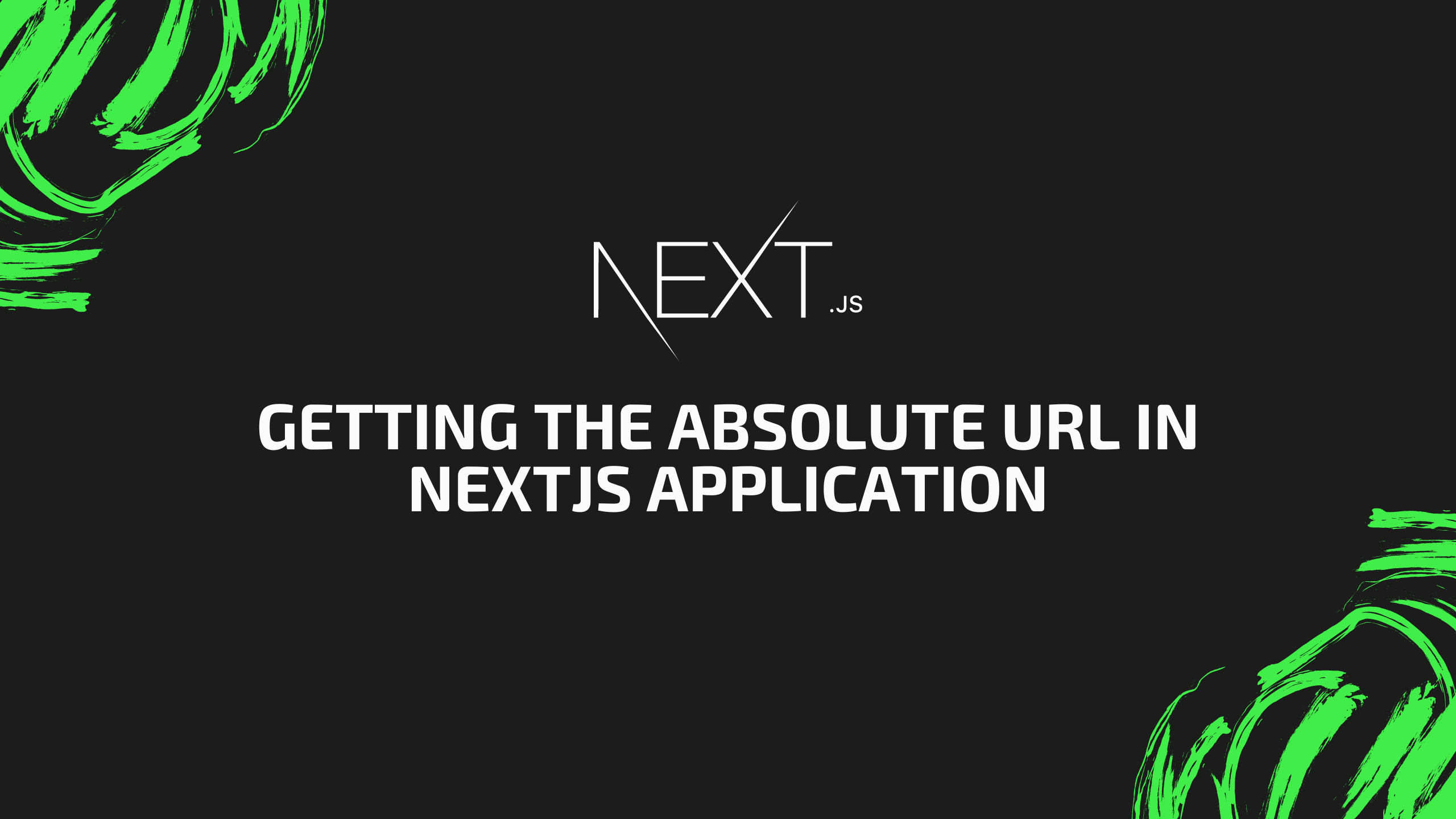
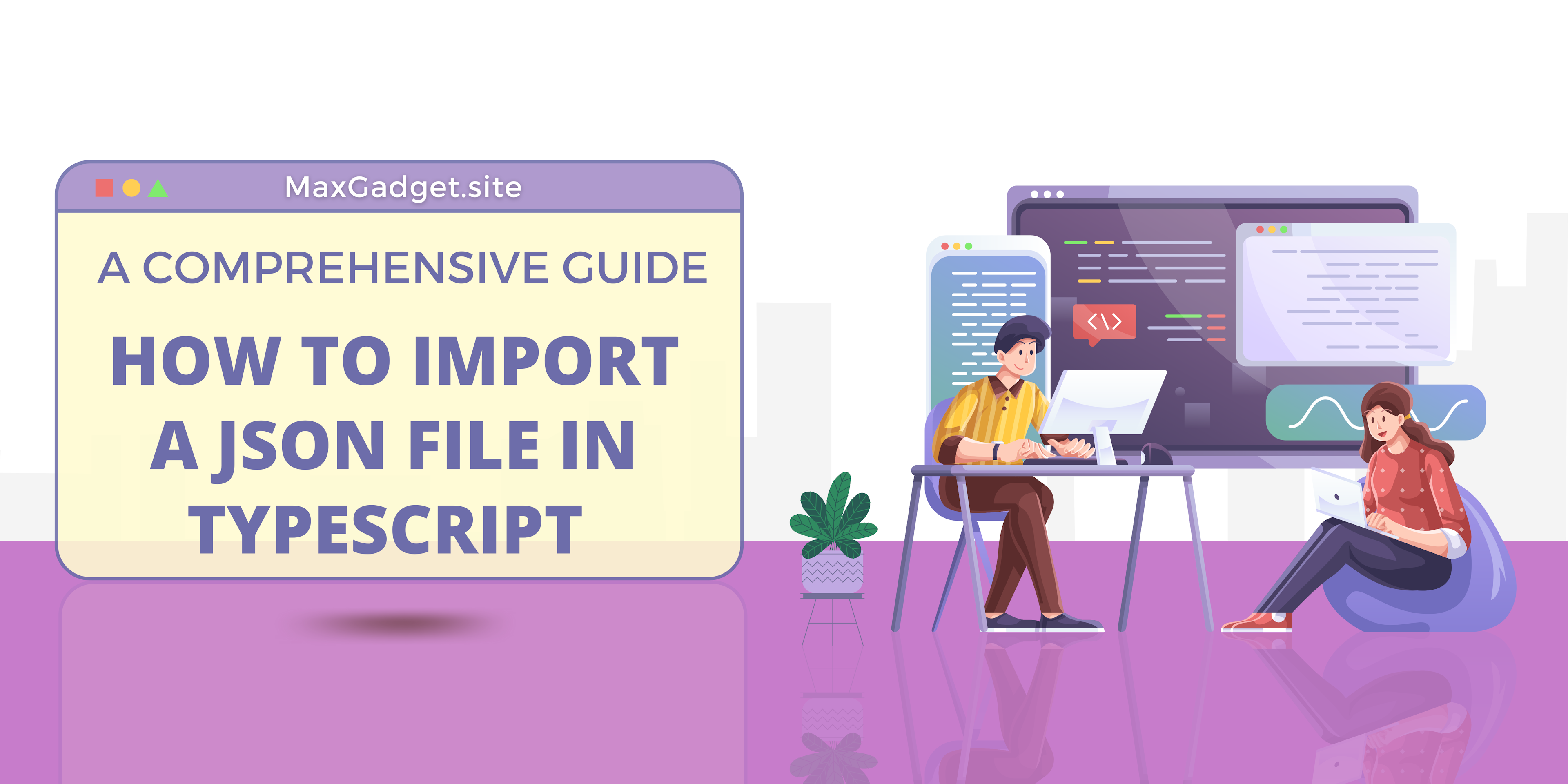
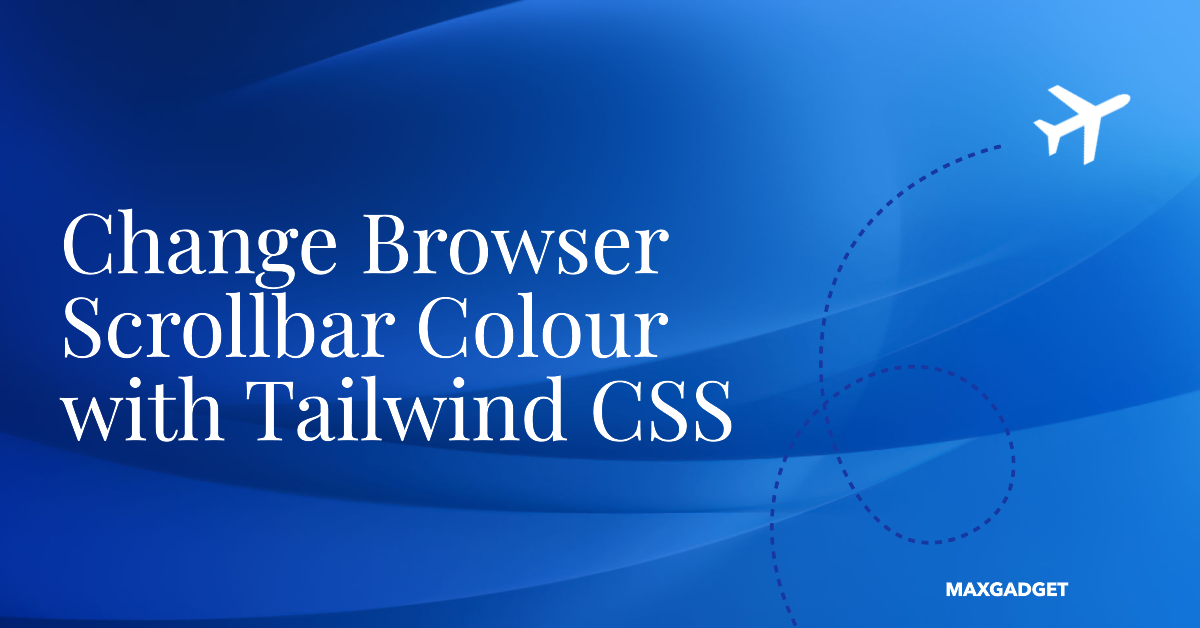
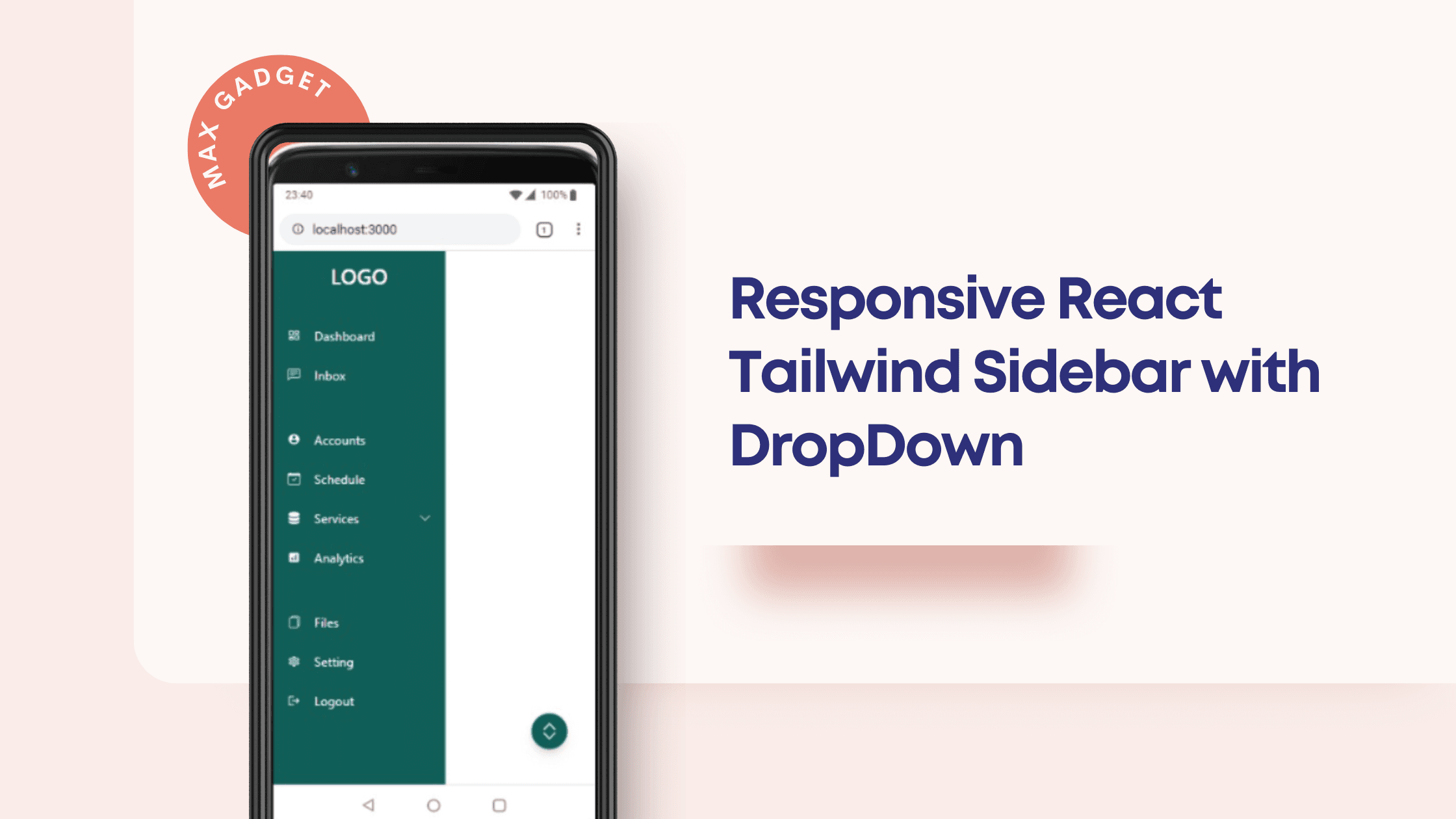