How to Import a JSON file in Typescript: A Comprehensive Guide

In the age of modern web development, working with JSON files is an essential skill. In this article, we'll explore importing a JSON file in Typescript. We will explore various techniques, including using Typescript's built-in capabilities, third-party libraries, and manual parsing.
Introduction to JSON and Typescript
Before diving into the specifics of importing JSON files in Typescript, it is crucial to understand what JSON (JavaScript Object Notation) and Typescript are and why they are important.
JSON is a lightweight data-interchange format that is easy to read and write. It is commonly used for exchanging data between a client and server or between different parts of an application.
Typescript is a statically-typed superset of JavaScript that compiles down to plain JavaScript. Typescript adds optional type-checking and other features to JavaScript, making it more robust and easier to maintain.
Using require() for Importing JSON files
The simplest way to import a JSON file in Typescript is using the require() function. This approach is compatible with CommonJS module systems and works seamlessly in a Node.js environment.
To use the require() function, follow these steps:
- Enable the 'resolveJsonModule' and 'esModuleInterop' compiler options in your 'tsconfig.json' file:
{
"compilerOptions": {
"resolveJsonModule": true,
"esModuleInterop": true
}
}
2. Import the JSON file using the require() function:
const data = require('./data.json');
With these steps, the JSON file is now available as a native JavaScript object, and you can access its properties as you normally would.
Importing JSON files with ES6 Modules
If you prefer using ES6 modules, Typescript also supports typescript import JSON files through the import statement. To do this, follow these steps:
- Enable the resolveJsonModule and esModuleInterop compiler options in your tsconfig.json file, as described in the previous section.
- Import the JSON file using the import statement:
import data from './data.json';
Now, you can use the imported JSON data as a regular JavaScript object.
Utilizing the JSON Module in Typescript
Typescript provides a built-in JSON module for working with JSON data. This module includes methods for parsing and stringifying JSON data, which can be useful when importing JSON typescript.
Here's how to use the JSON module to import a JSON file:
- Read the JSON file using the fs module in Node.js:
import fs from 'fs';
const jsonString = fs.readFileSync('./data.json', 'utf-8');
2. Parse the JSON string using the JSON.parse() method:
const data = JSON.parse(jsonString);
With this approach, the JSON file is imported and parsed, allowing you to access its properties as you would with a regular JavaScript object.
Leveraging Third-Party Libraries
There are several third-party libraries available that can help you import JSON files in Typescript. One popular library is axios, which is a promise-based HTTP client for the browser and Node.js.
Here's how to use axios to import a JSON file:
- Install the axios library:
npm install axios
2. Import axios and use it to fetch the JSON file:
import axios from 'axios';
async function fetchData() {
try {
const response = await axios.get('./data.json');
const data = response.data;
console.log(data);
} catch (error) {
console.error(error);
}
}
fetchData();
Using axios, you can easily import JSON files from both local files and remote URLs, making it a versatile solution for various use cases.
Manually Parsing JSON Files
If you need more control over the process of importing and parsing JSON files, you can create your own custom function for this purpose. This approach can be useful when dealing with large JSON files or when you need to perform additional data manipulation.
Here's an example of a custom function to import and parse a JSON file:
import fs from 'fs';
function importJsonFile(filePath: string): object {
const fileContent = fs.readFileSync(filePath, 'utf-8');
try {
const jsonData = JSON.parse(fileContent);
return jsonData;
} catch (error) {
throw new Error(`Error parsing JSON file: ${error.message}`);
}
}
const data = importJsonFile('./data.json');
console.log(data);
This custom function provides you with the flexibility to handle JSON files as needed, depending on your project's requirements.
Choosing the Best Method for Your Project
The choice of which method to use for importing JSON files in Typescript depends on several factors, such as:
- Your project's environment (Node.js or browser)
- The module system you are using (CommonJS or ES6 modules)
- Whether you need additional features provided by third-party libraries
- The level of control and customization required for handling JSON files
Each method has its pros and cons, so it is important to carefully consider your project's needs before choosing the most suitable approach.
Conclusion
In this comprehensive guide, we have explored various methods for importing JSON files in Typescript. From using the require() function and ES6 module imports to leveraging third-party libraries and creating custom functions, there are numerous options available to suit different project requirements.
By understanding these methods and their advantages, you can make an informed decision about the best way to import JSON files in your Typescript project, ultimately improving the efficiency and maintainability of your code.
Frequently Asked Questions
1. Can I import JSON files directly in the browser?
While you cannot import JSON files directly using the import statement in a browser environment, you can use third-party libraries like axios or the built-in fetch() function to fetch JSON data from a server or local file.
2. Do I need to install additional packages to import JSON files in Typescript?
No, Typescript natively supports importing JSON files through the require() function and ES6 module imports. However, third-party libraries like axios can be useful for certain use cases, such as fetching JSON files from remote servers.
3. Is there a performance difference between the various methods of importing JSON files in Typescript?
Performance differences between the methods are generally minimal and may not have a significant impact on most applications. However, when working with large JSON files or dealing with a high number of requests, using third-party libraries like axios or creating custom functions can provide better performance and error handling.
4. Can I import JSON files with a dynamic file path in Typescript?
Yes, you can use typescript import JSON file with a dynamic file path by using the fs module in Node.js or the fetch() function in the browser. For instance, you can read and parse JSON files based on user input or other variables in your application.
5. How do I handle errors when importing JSON files in Typescript?
Error handling is essential when working with JSON files, as it helps ensure the reliability of your application. You can handle errors by using try-catch blocks when parsing JSON data, checking for the existence of the file before reading, or implementing error handling in custom functions or third-party libraries like axios. By properly handling errors, you can provide better user experiences and prevent application crashes due to invalid JSON data.
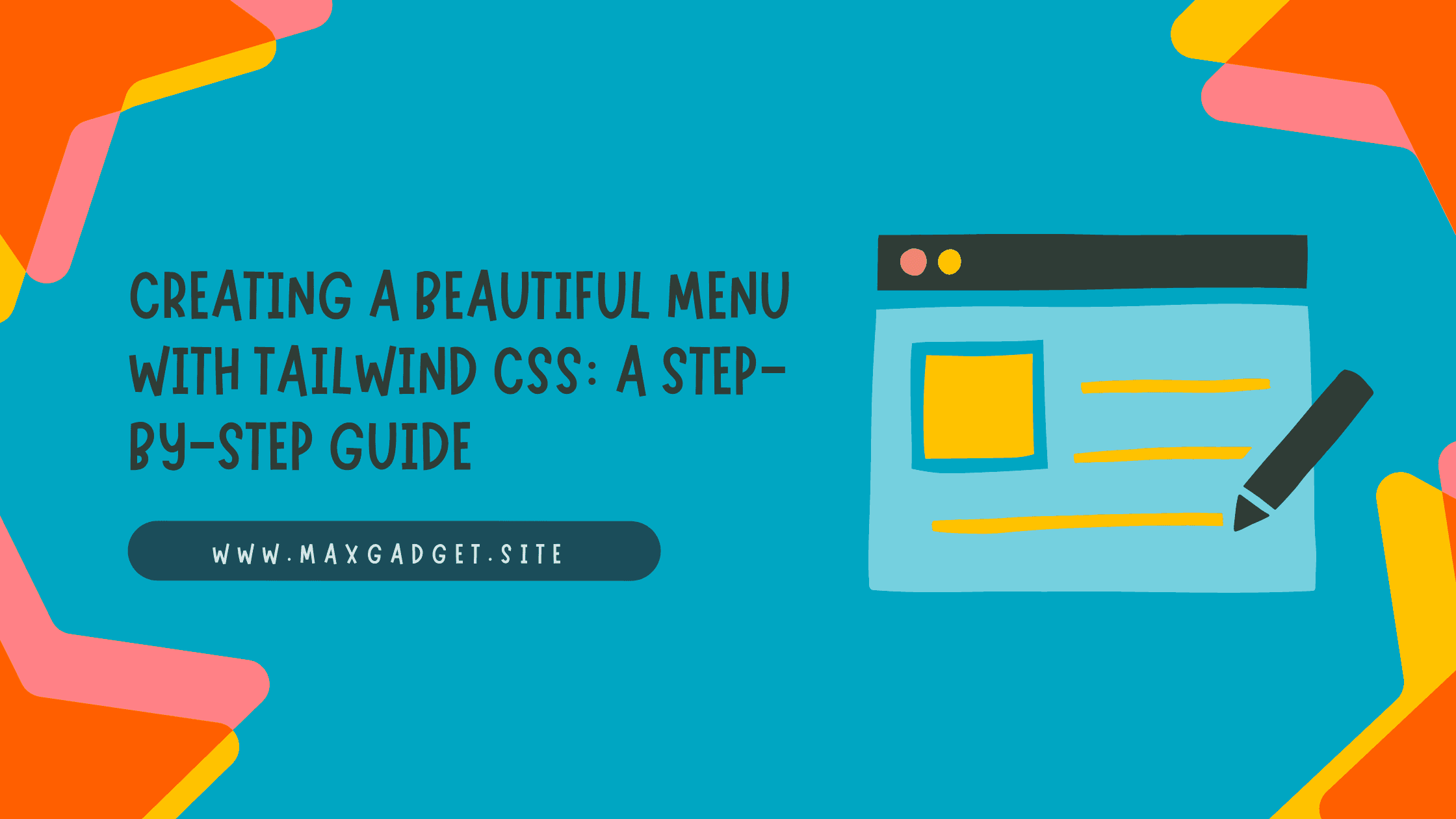
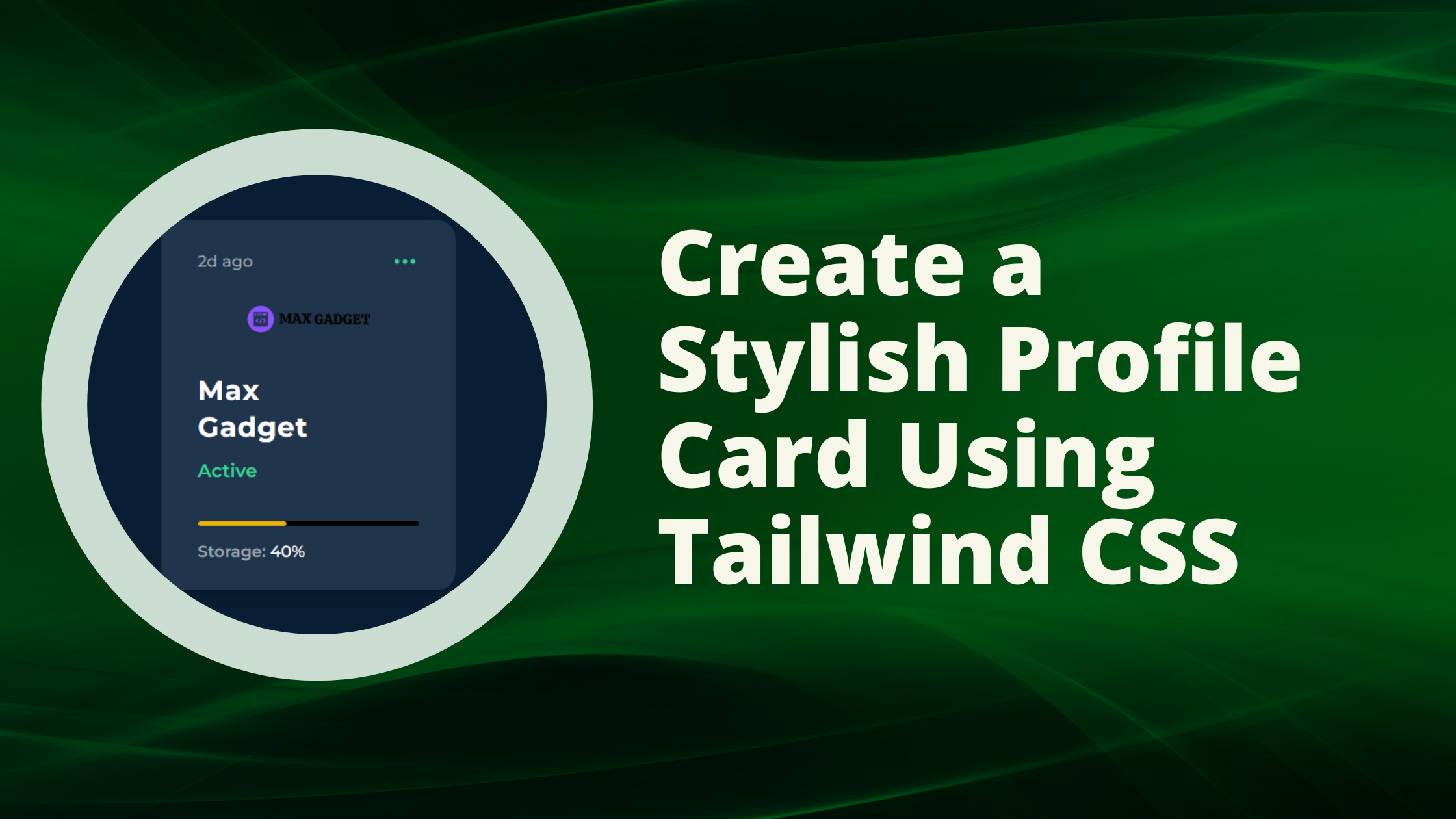
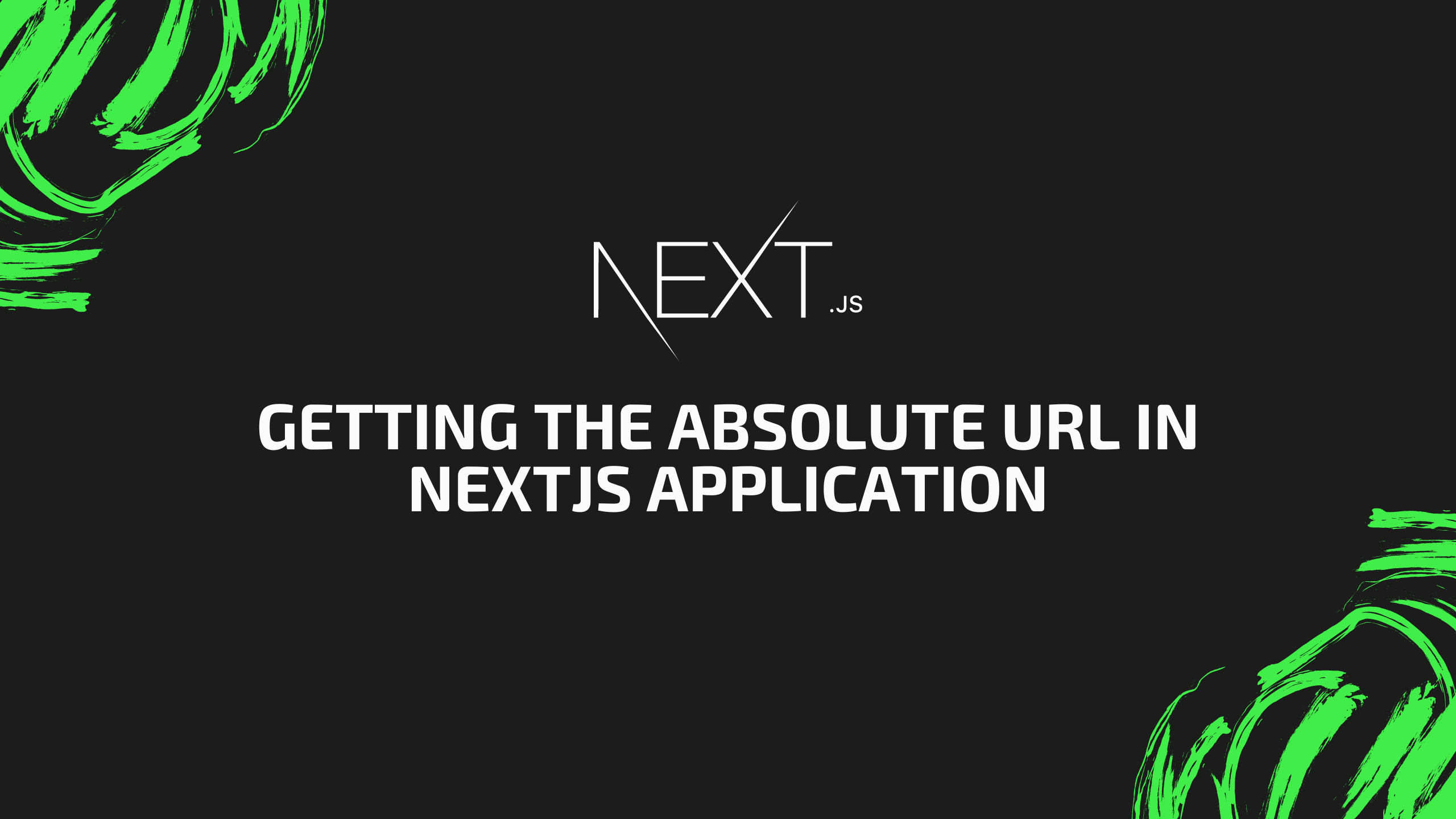
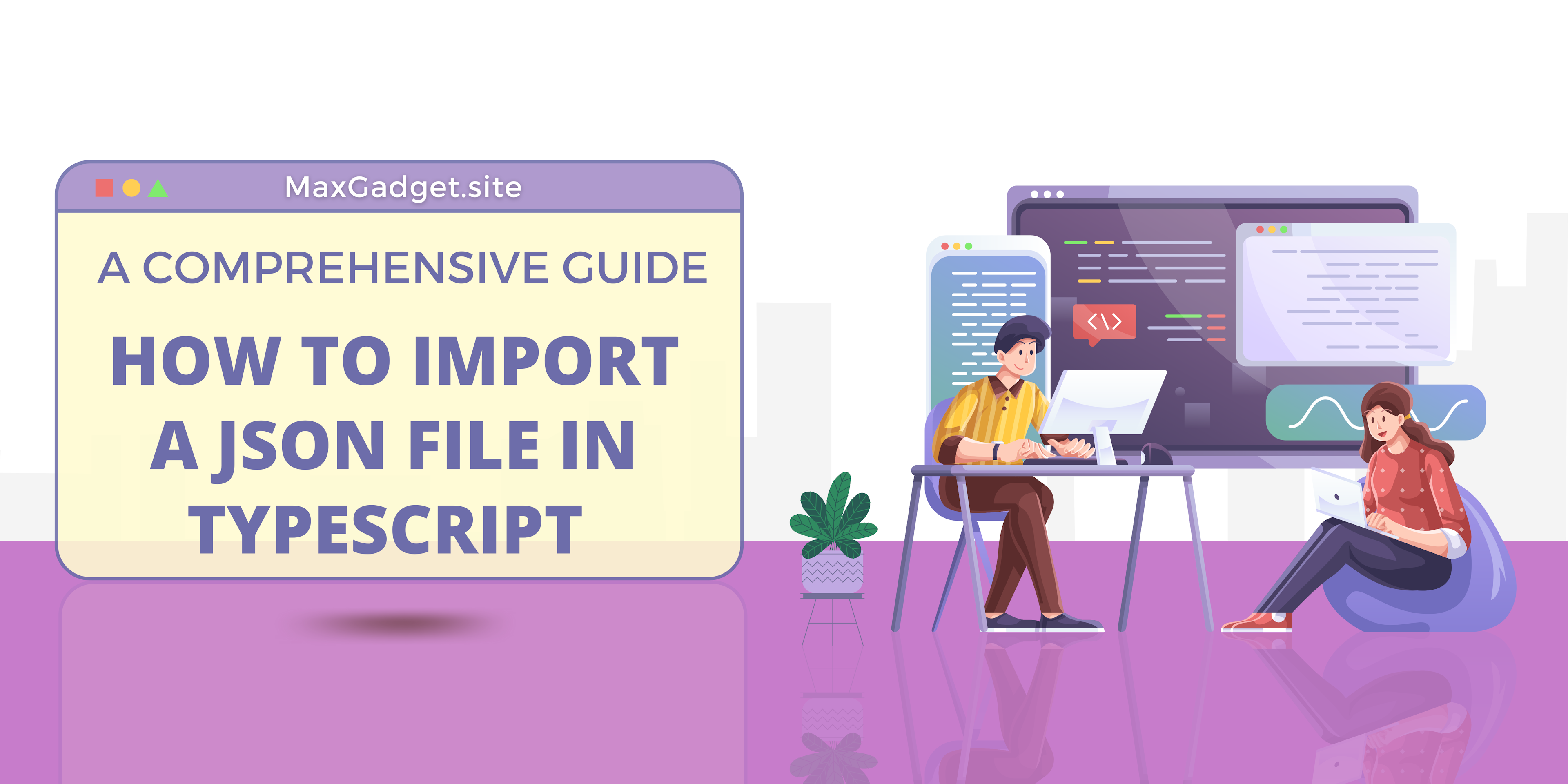
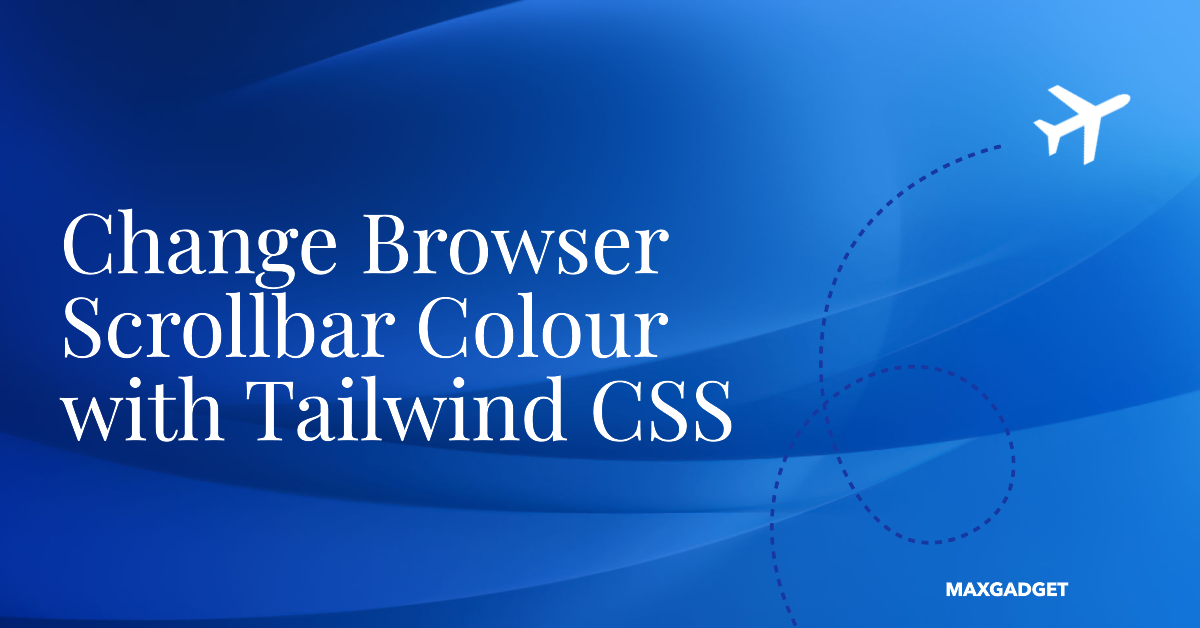
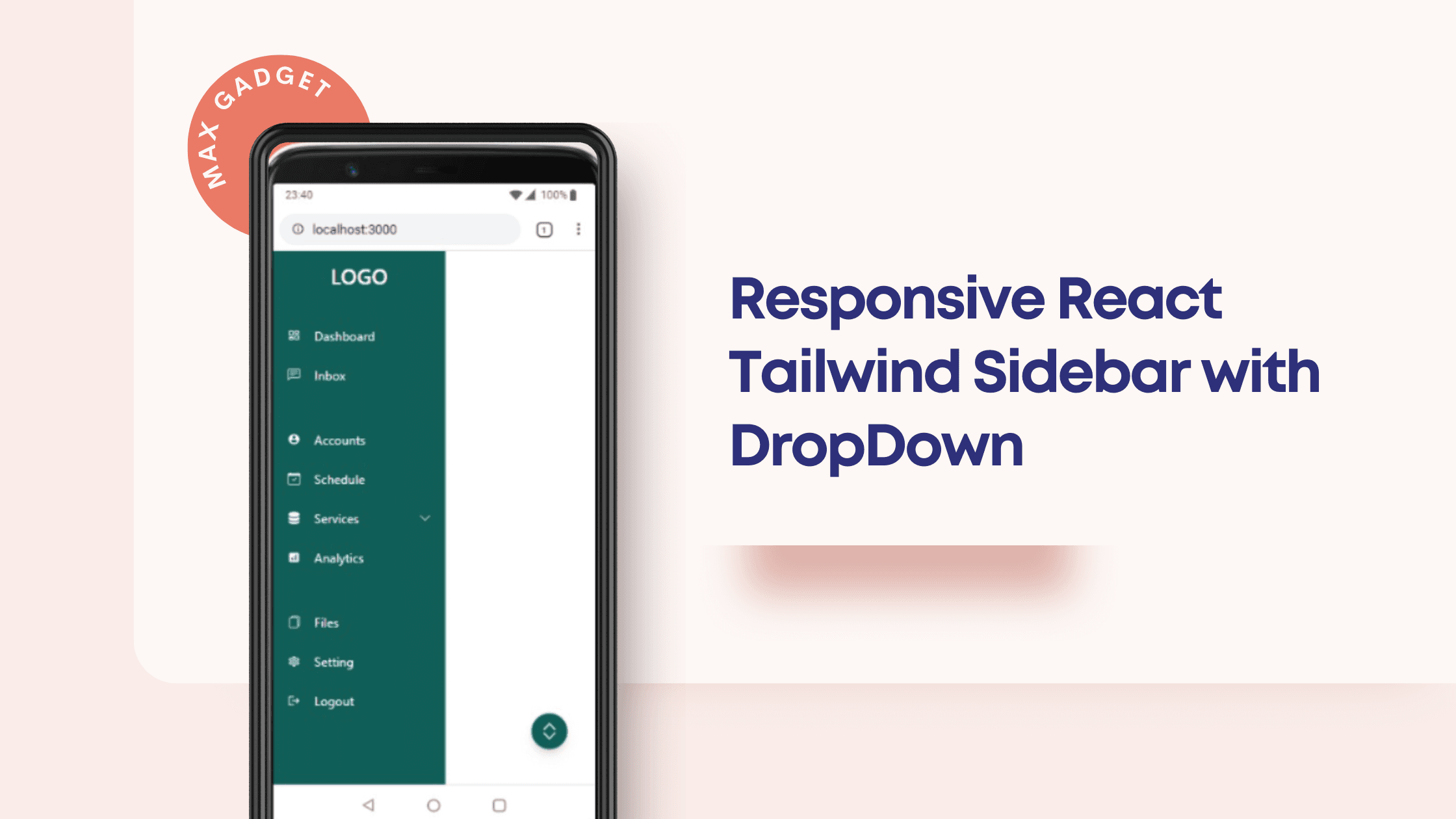