How to use For Loop inside React JSX: Guide

If You are wondering about running for loop inside react JSX and thinking if it’s even possible, then the answer is Yes. You can use for loop inside JSX, but you may not see people doing it. It is because it hampers the performance of the overall code. You can use Map function instead of the for loop. But if you are interested in doing it with for loop then here is how you can do it.
Let’s create an Array with employee names that we will use to iterate through the for loop in JSX.
const employees = ["Abhinav", "Kevin", "Shophie", "Gaurav"];
Now, if we try the following code, it will not work. But, why?
import "./styles.css";
const employees = ["Abhinav", "Kevin", "Shophie", "Gaurav"];
export default function App() {
return <div className="App">{
for (let i = 0; i < employees.length; i++) {
employees[i]
}
</div>;
}
If we need to run normal JavaScript within JSX then we must use {}. Curly braces are used to run an expression. And an expression can be anything like callback function, method call, one line function or even ternary operators.
How to use For Loop in React JSX:
We can create a separate function named EmployeeName and use the for loop to iterate the names from the array. Once done, we can call the function EmployeeName() inside the curly braces.
import "./styles.css";
const employees = ["Abhinav", "Kevin", "Shophie", "Gaurav"];
export default function App() {
const employeeName = () => {
const row = [];
for (let i = 0; i < employees.length; i++) {
row.push(<p key={i}>{employees[i]}</p>);
}
return row;
};
return <div className="App">
{employeeName()}
</div>;
}
The above example will definitely work, but if you don’t want to do this. You can use the second method.
Using the Map method to Loop inside the React JSX
Map method is the most common and feasible way to do iteration inside the jsx. You don’t need to create any different function for this. Just use .map() function and iterate through the array like the example below.
import "./styles.css";
const employees = ["Abhinav", "Kevin", "Shophie", "Gaurav"];
export default function App() {
return <div className="App">
{employees.map((employee)=>{
return (
<h1>{employee}</h1>)
})}
</div>;
}
So, this is how you can use for loop in React JSX and map in react JSX. You can get the code from this link. Feel free to comment if you have any doubts regarding the same.
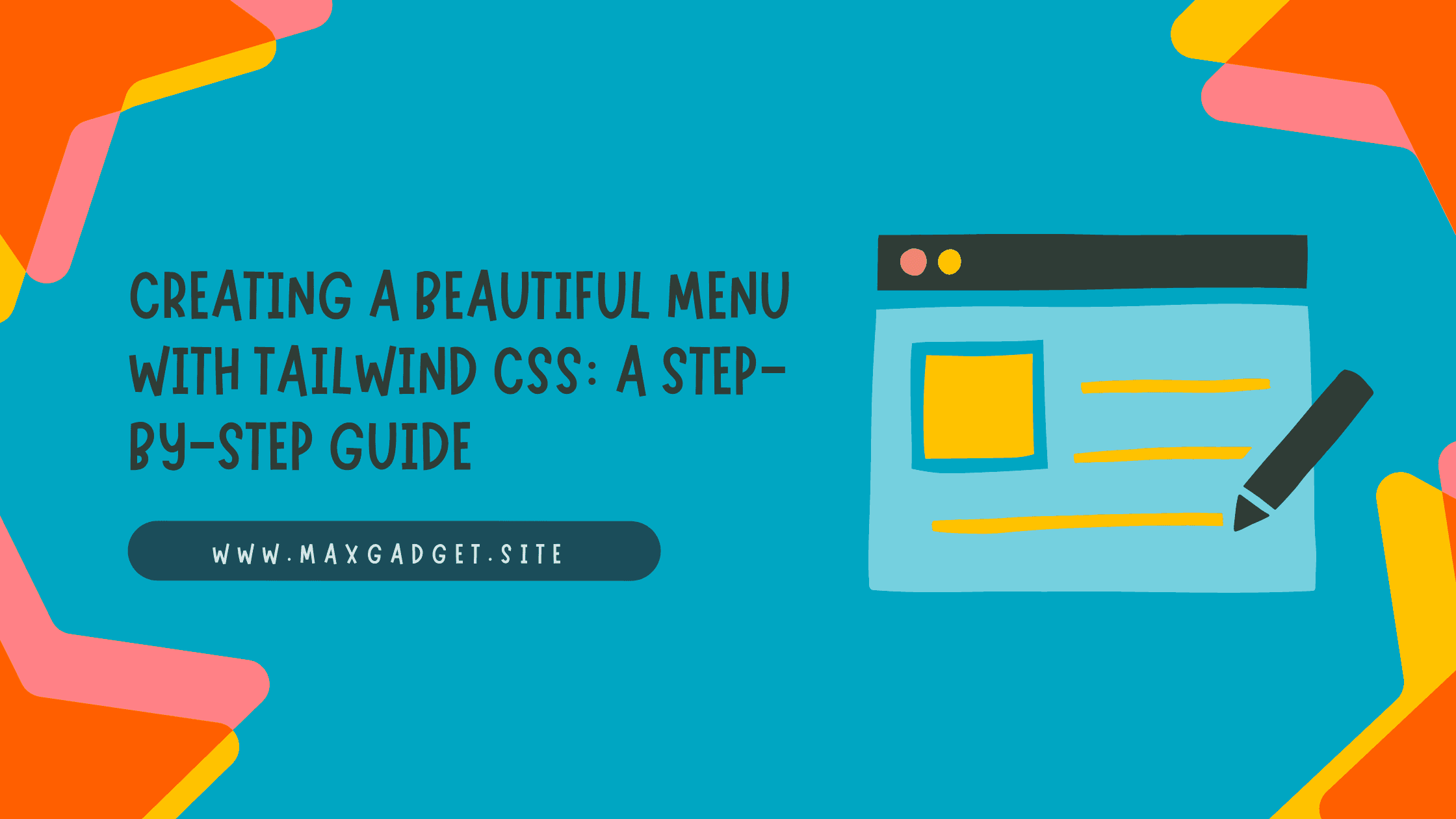
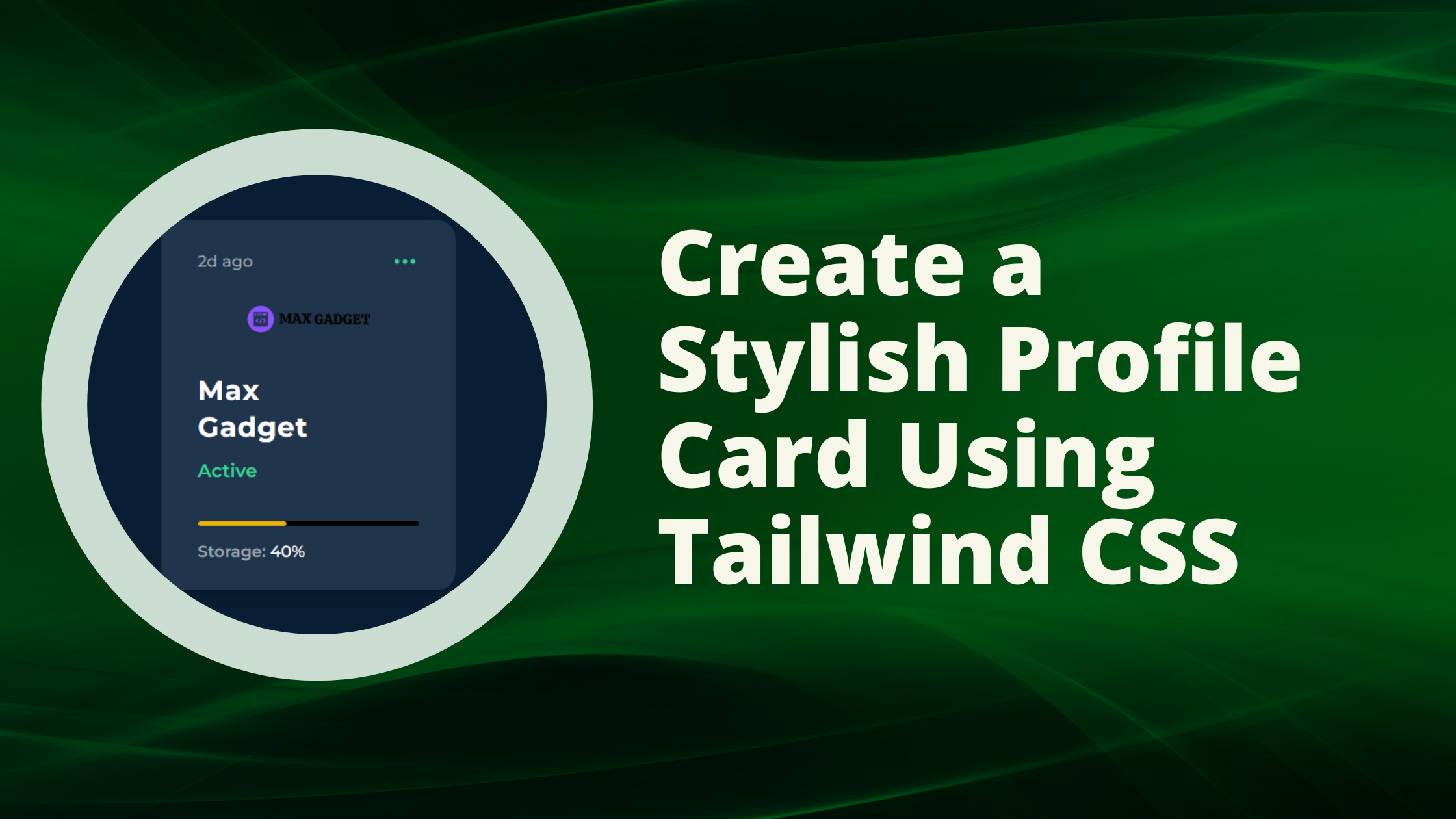
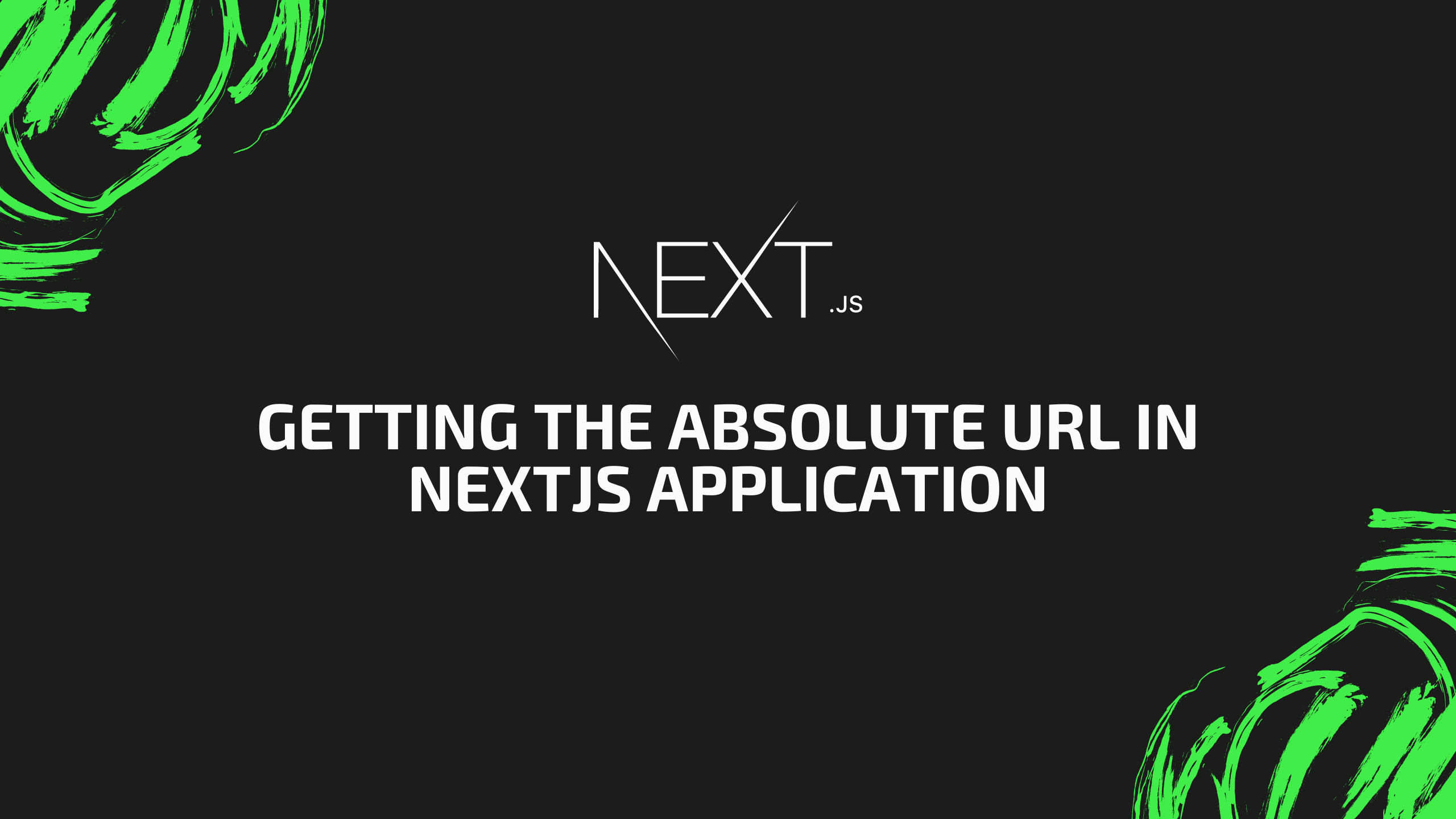
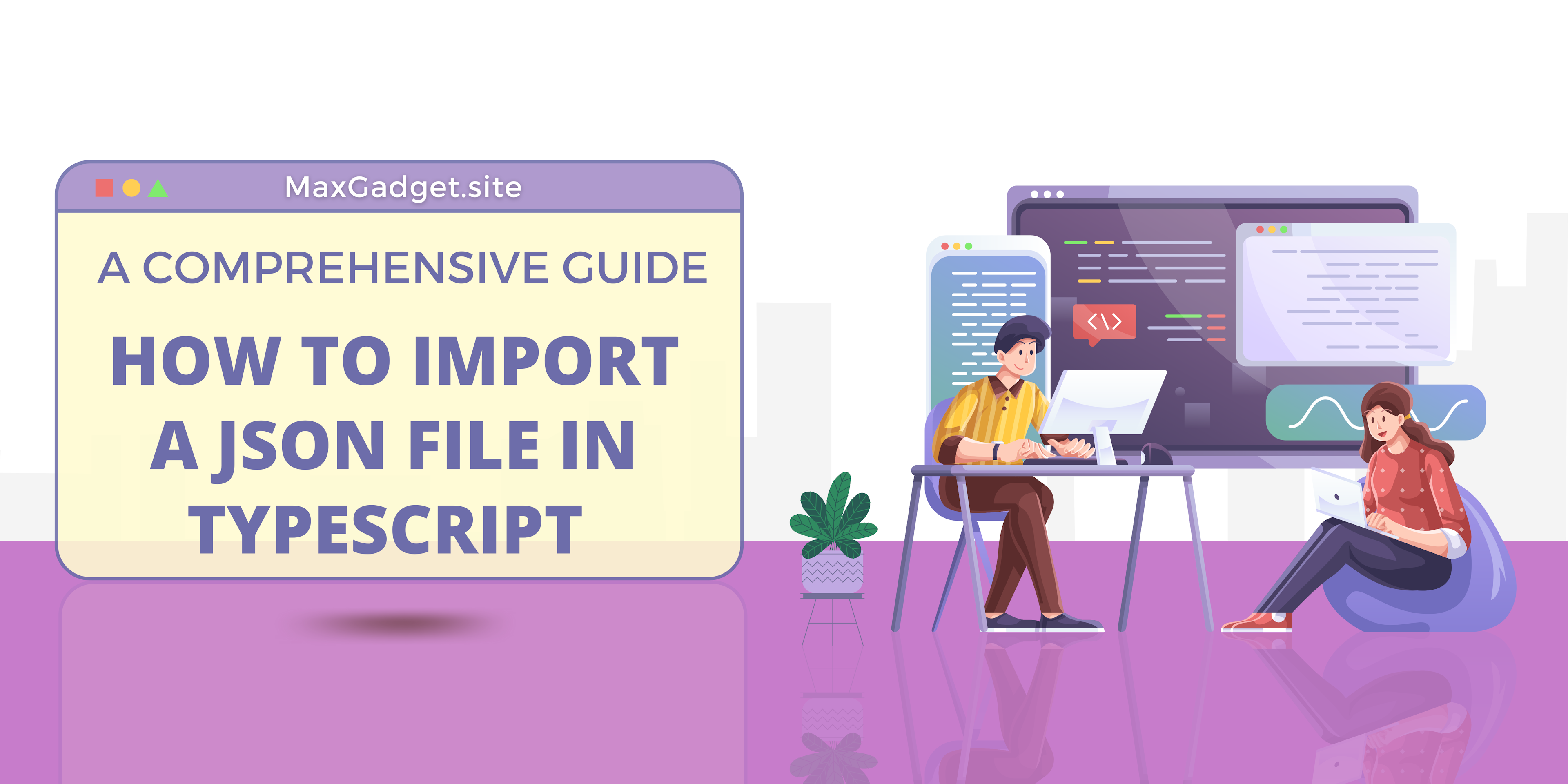
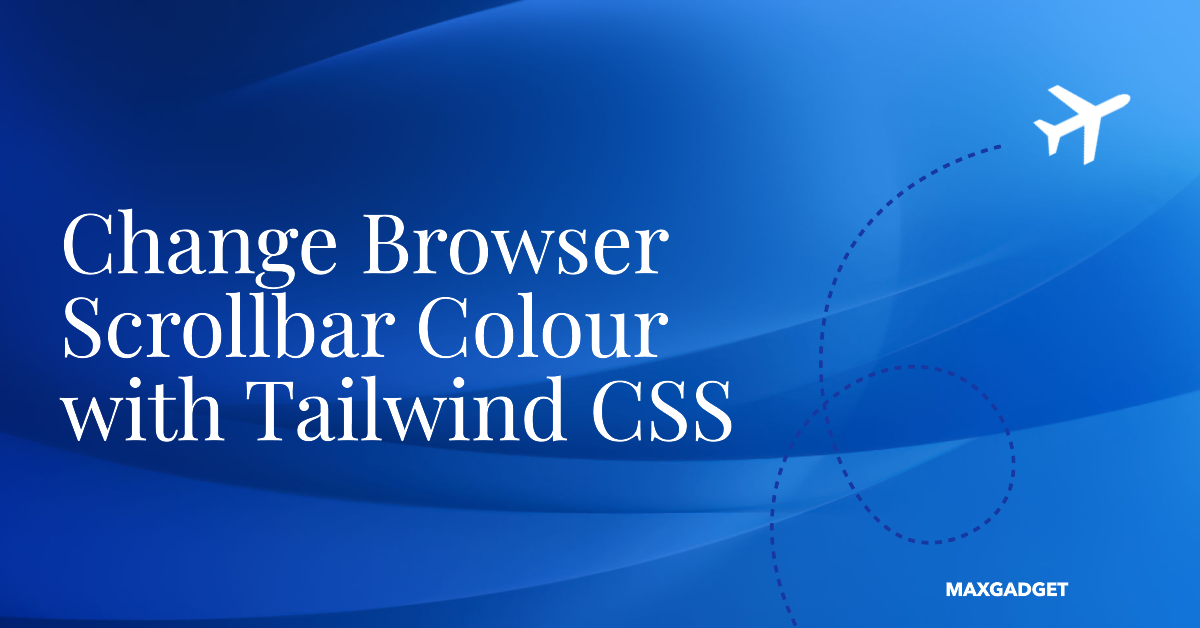
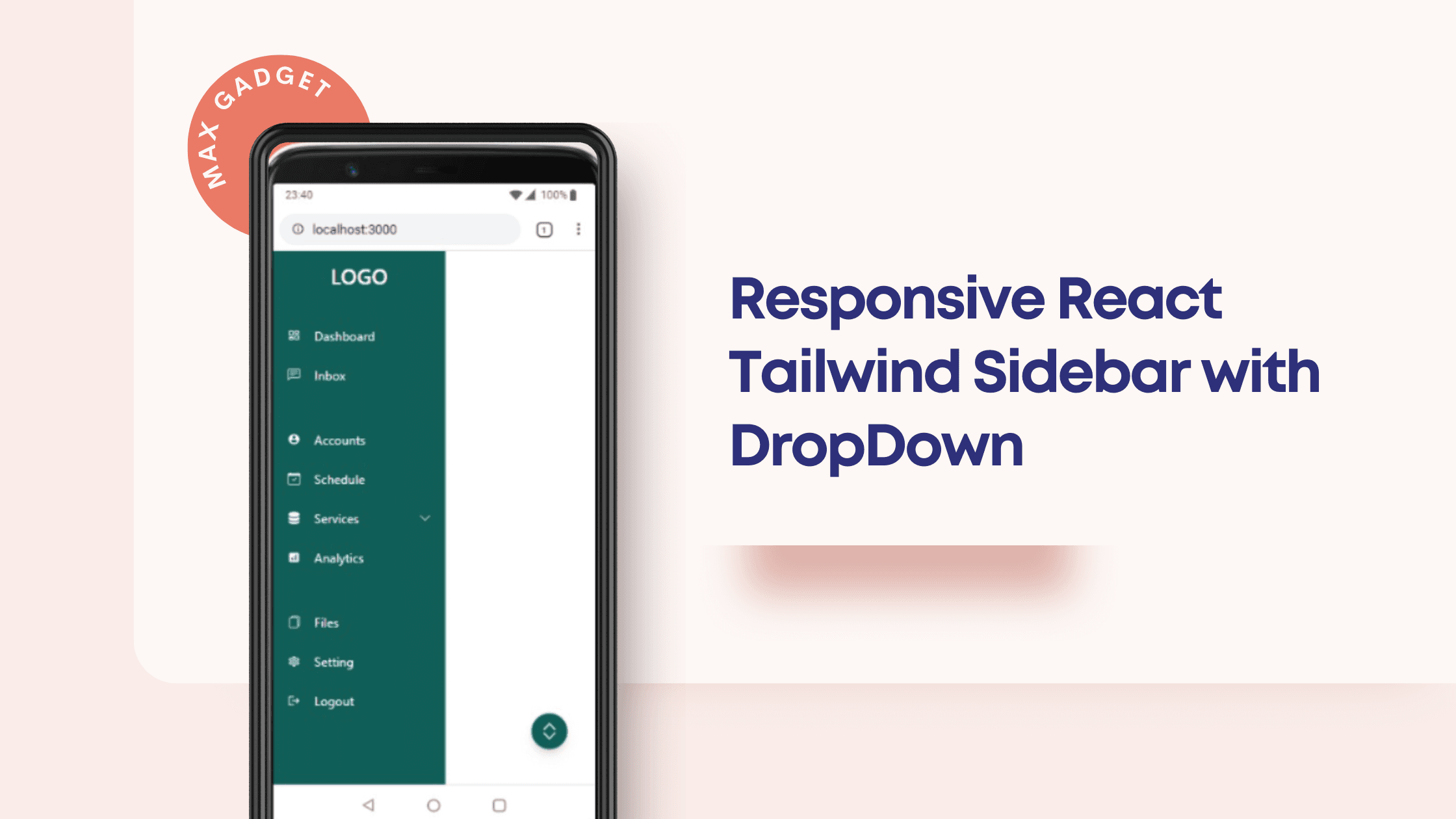